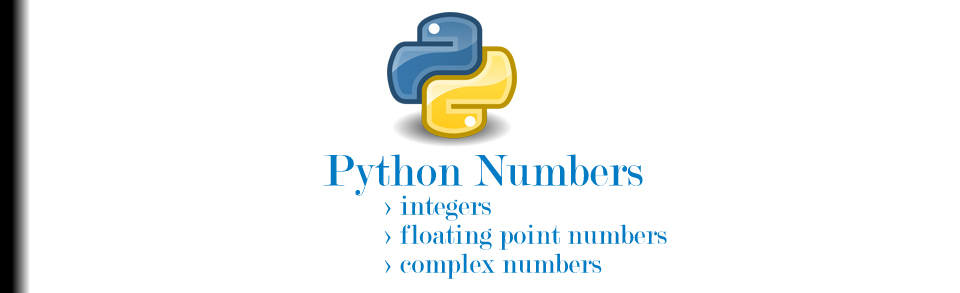
Python – Number, Data sorts shop numeric values. They are immutable records kinds, approach that converting the value of quite a number information type results in a newly allotted object.
Number gadgets are created while you assign a value to them. For instance −
var1 = 1
var2 = 10
You also can delete the connection with more than a few object with the aid of the use of the del declaration. The syntax of the del statement is −
del var1[,var2[,var3[....,varN]]]]
You can delete a single item or a couple of items via using the del assertion. For example −
del var
del var_a, var_b
Python supports four special numerical kinds −
- int (signed integers) − They are frequently called just integers or ints, are nice or negative whole numbers with no decimal factor.
- Lengthy (lengthy integers ) − Also called longs, they’re integers of limitless size, written like integers and followed by means of an uppercase or lowercase L.
- Flow (floating point real values) − Also referred to as floats, they represent real numbers and are written with a decimal factor dividing the integer and fractional elements. Floats can also be in medical notation, with E or e indicating the power of 10 (2.5e2 = 2.5 x 102 = 250).
- Complex (complex numbers) − are of the form a + bJ, wherein a and b are floats and J (or j) represents the square root of -1 (which is an imaginary quantity). The actual part of the number is a, and the imaginary element is b. Complex numbers are not used lots in Python programming.
Examples
Here are some examples of numbers
int | long | float | complex |
---|---|---|---|
10 | 51924361L | 0.0 | 3.14j |
100 | -0x19323L | 15.20 | 45.j |
-786 | 0122L | -21.9 | 9.322e-36j |
080 | 0xDEFABCECBDAECBFBAEL | 32.3+e18 | .876j |
-0490 | 535633629843L | -90. | -.6545+0J |
-0x260 | -052318172735L | -32.54e100 | 3e+26J |
0x69 | -4721885298529L | 70.2-E12 | 4.53e-7j |
- Python lets in you to use a lowercase L with long, but it’s far endorsed which you use most effective an uppercase L to keep away from confusion with the #1. Python presentations lengthy integers with an uppercase L.
- A complicated wide variety includes an ordered pair of real floating point numbers denoted through a + bj, wherein a is the real component and b is the imaginary a part of the complex range.
Number Type Conversion
Python converts numbers internally in an expression containing mixed kinds to a not unusual kind for evaluation. But from time to time, you need to coerce more than a few explicitly from one type to some other to fulfill the requirements of an operator or function parameter.
- Type int(x) to transform x to a undeniable integer.
- Type lengthy(x) to convert x to a protracted integer.
- Type glide(x) to convert x to a floating-point wide variety.
- Type complex(x) to transform x to a complicated range with real part x and imaginary component zero.
- Type complex(x, y) to convert x and y to a complex wide variety with real part x and imaginary component y. X and y are numeric expressions
Mathematical Functions
Python consists of following functions that perform mathematical calculations.
Sr.No. | Function & Returns ( description ) |
---|---|
1 | abs(x)The absolute value of x: the (positive) distance between x and zero. |
2 | ceil(x)The ceiling of x: the smallest integer not less than x |
3 | cmp(x, y)-1 if x < y, 0 if x == y, or 1 if x > y |
4 | exp(x)The exponential of x: ex |
5 | fabs(x)The absolute value of x. |
6 | floor(x)The floor of x: the largest integer not greater than x |
7 | log(x)The natural logarithm of x, for x> 0 |
8 | log10(x)The base-10 logarithm of x for x> 0. |
9 | max(x1, x2,…)The largest of its arguments: the value closest to positive infinity |
10 | min(x1, x2,…)The smallest of its arguments: the value closest to negative infinity |
11 | modf(x)The fractional and integer parts of x in a two-item tuple. Both parts have the same sign as x. The integer part is returned as a float. |
12 | pow(x, y)The value of x**y. |
13 | round(x [,n])x rounded to n digits from the decimal point. Python rounds away from zero as a tie-breaker: round(0.5) is 1.0 and round(-0.5) is -1.0. |
14 | sqrt(x)The square root of x for x > 0 |
Random Number Functions
Python – Number, Random numbers are used for games, simulations, testing, safety, and privateness applications. Python consists of following functions that are typically used.
Sr.No. | Function & Description |
---|---|
1 | choice(seq)A random item from a list, tuple, or string. |
2 | randrange ([start,] stop [,step])A randomly selected element from range(start, stop, step) |
3 | random()A random float r, such that 0 is less than or equal to r and r is less than 1 |
4 | seed([x])Sets the integer starting value used in generating random numbers. Call this function before calling any other random module function. Returns None. |
5 | shuffle(lst)Randomizes the items of a list in place. Returns None. |
6 | uniform(x, y)A random float r, such that x is less than or equal to r and r is less than y |
Python – Number, Trigonometric Functions
Python includes following functions that carry out trigonometric calculations.
Sr.No. | Function & Description |
---|---|
1 | acos(x)Return the arc cosine of x, in radians. |
2 | asin(x)Return the arc sine of x, in radians. |
3 | atan(x)Return the arc tangent of x, in radians. |
4 | atan2(y, x)Return atan(y / x), in radians. |
5 | cos(x)Return the cosine of x radians. |
6 | hypot(x, y)Return the Euclidean norm, sqrt(x*x + y*y). |
7 | sin(x)Return the sine of x radians. |
8 | tan(x)Return the tangent of x radians. |
9 | degrees(x)Converts angle x from radians to degrees. |
10 | radians(x)Converts angle x from degrees to radians. |
Python – Number, Mathematical Constants
The module also defines two mathematical constants −
Sr.No. | Constants & Description |
---|---|
1 | piThe mathematical constant pi. |
2 | eThe mathematical constant e. |