Starting C++ Classes
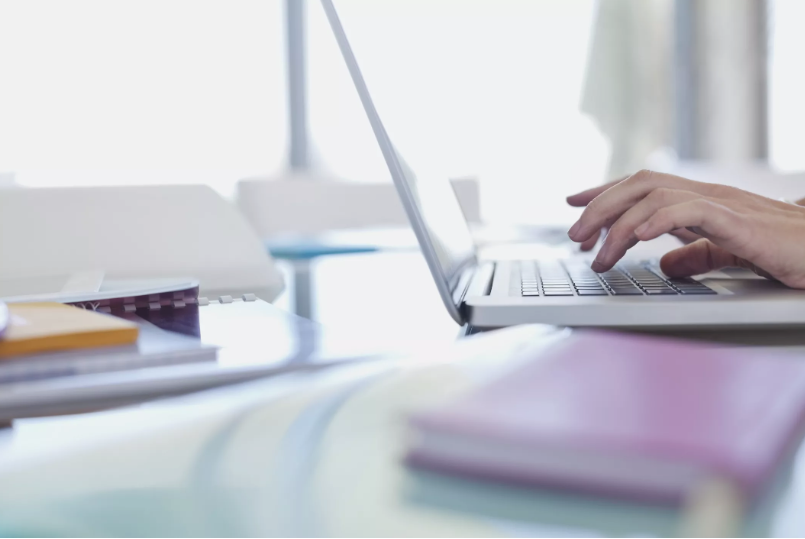
C++ Classes,Objects are the most important distinction between C++ and C. One of the earliest names for C++ turned into C with Classes.
C++ Classes,Classes and Objects
C++ Classes,A magnificence is a definition of an item. It’s a type just like int. A magnificence resembles a struct with just one difference: all struct contributors are public by way of default. All instructions contributors are personal.
Remember—a class is a kind, and an object of this class is just a variable.
Before we are able to use an item, it must be created. The handiest definition of a class is:
class name {
// members
}
This instance class under fashions a simple e book. Using OOP lets you abstract the trouble and reflect onconsideration on it and not just arbitrary variables.
// example one
#include
#include
class Book
{
int PageCount;
int CurrentPage;
public:
Book( int Numpages) ; // Constructor
~Book(){} ; // Destructor
void SetPage( int PageNumber) ;
int GetCurrentPage( void ) ;
};
Book::Book( int NumPages) {
PageCount = NumPages;
}
void Book::SetPage( int PageNumber) {
CurrentPage=PageNumber;
}
int Book::GetCurrentPage( void ) {
return CurrentPage;
}
int main() {
Book ABook(128) ;
ABook.SetPage( 56 ) ;
std::cout << "Current Page " << ABook.GetCurrentPage() << std::endl;
return 0;
}
All the code from magnificence e-book down to the int Book::GetCurrentPage(void) characteristic is part of the magnificence. The principal() feature is there to make this a runnable utility.
C++ Classes,Understanding the Book Class
C++ ClassesIn the primary() characteristic a variable ABook of type Book is created with the price 128. As quickly as execution reaches this point, the item ABook is constructed. On the following line the technique ABook.SetPage() is referred to as and the fee fifty six assigned to the object variable ABook.CurrentPage. Then cout outputs this fee by way of calling the Abook.GetCurrentPage() method.
When execution reaches the return 0; the ABook object is not wanted via the application. The compiler generates a call to the destructor.
Declaring Classes
Everything among Class Book and the is the magnificence declaration. This elegance has non-public contributors, both of type int. These are private because the default get right of entry to to elegance participants is private.
The public: directive tells the compiler that accesses from here on is public. Without this, it’d nonetheless be personal and prevent the 3 strains in the main() function from gaining access to Abook participants. Try commenting the general public: line out and recompiling to see the ensuing compile errors.
This line beneath pronounces a Constructor. This is the feature referred to as when the object is first created.
Book( int Numpages) ; // Constructor
It is called from the line
Book ABook(128) ;
This creates an object referred to as ABook of type Book and calls the Book() feature with the parameter 128.
More About the Book Class
In C++, the constructor continually has the identical name as the magnificence. The constructor is referred to as whilst the object is created and is where you need to placed your code to initialize the object.
In Book The next line after the constructor the destructor. This has the same name because the constructor however with a ~ (tilde) in front of it. During the destruction of an item, the destructor is known as to tidy up the object and make certain that resources which include memory and report deal with utilized by the item are released.
Remember—a class xyz has a constructor characteristic xyz() and destructor function ~xyz(). Even in case you do not claim, the compiler will silently add them.
The destructor is usually known as whilst the object is terminated. In this case, the item is implicitly destroyed while it is going out of scope. To see this, modify the destructor announcement to this:
~Book(){ std::cout << "Destructor called";} ; // Destructor
This is an inline function with code in the declaration. Another way to inline is adding the word inline
inline ~Book() ; // Destructor
and add the destructor as a function like this.
inline Book::~Book ( void ) {
std::cout << "Destructor called";
}
Inline functions are hints to the compiler to generate greater green code. They should best be used for small capabilities, but if used in suitable locations—which include internal loops—could make a significant distinction in performance.
Writing Class Methods
Best exercise for items is to make all facts non-public and access it thru functions referred to as accessor functions. SetPage() and GetCurrentPage() are the 2 features used to access the item variable CurrentPage.
Change the class statement to struct and recompile. It should still collect and run successfully. Now the 2 variables PageCount and CurrentPage are publicly on hand. Add this line after the Book ABook(128), and it’s going to bring together.
ABook.PageCount =9;
If you convert struct again to class and recompile, that new line will no longer compile as PageCount is now private again.
The :: Notation
After the frame of Book Class declaration, there are the four definitions of the member capabilities. Each is described with the Book:: prefix to become aware of it as belonging to that elegance. :: is called the scope identifier. It identifies the characteristic as being part of the magnificence. This is apparent inside the elegance declaration however no longer outside it.
If you have declared a member characteristic in a category, you ought to offer the frame of the characteristic in this way. If you desired the Book magnificence to be utilized by different documents then you might flow the statement of book into a separate header document, possibly called ebook.H. Any other file ought to then encompass it with
#include "book.h"
Inheritance and Polymorphism
This example will demonstrate inheritance. This is a two class application with one class derived from another.
#include
#include
class Point
{
int x,y;
public:
Point(int atx,int aty ) ; // Constructor
inline virtual ~Point() ; // Destructor
virtual void Draw() ;
};
class Circle : public Point {
int radius;
public:
Circle(int atx,int aty,int theRadius) ;
inline virtual ~Circle() ;
virtual void Draw() ;
};
Point ::Point(int atx,int aty) {
x = atx;
y = aty;
}
inline Point::~Point ( void ) {
std::cout << "Point Destructor called";
}
void Point::Draw( void ) {
std::cout << "Point::Draw point at " << x << " " << y << std::endl;
}
Circle::Circle(int atx,int aty,int theRadius) : Point(atx,aty) {
radius = theRadius;
}
inline Circle::~Circle() {
std::cout << "Circle Destructor called" << std::endl;
}
void Circle::Draw( void ) {
Point::Draw() ;
std::cout << "circle::Draw point " << " Radius "<< radius << std::endl;
}
int main() {
Circle ACircle(10,10,5) ;
ACircle.Draw() ;
return 0;
}
The instance has two instructions, Point and Circle, modeling a factor and a circle. A Point has x and y coordinates. The Circle elegance is derived from the Point magnificence and adds a radius. Both training consist of a Draw() member function. To preserve this example brief the output is simply textual content.
Inheritance
The magnificence Circle is derived from the Point class. This is accomplished in this line:
class Circle : Point {
Because it is derived from a base class (Point), Circle inherits all the class members.
Point(int atx,int aty ) ; // Constructor
inline virtual ~Point() ; // Destructor
virtual void Draw() ;
Circle(int atx,int aty,int theRadius) ;
inline virtual ~Circle() ;
virtual void Draw() ;
Think of the Circle magnificence as the Point magnificence with an extra member (radius). It inherits the bottom magnificence Member capabilities and private variables x and y.
It can not assign or use those besides implicitly because they’re personal, so it has to do it thru the Circle constructor’s Initializer listing. This is something you need to receive as is for now. I’ll come returned to initializer lists in a destiny tutorial.
In the Circle Constructor, before theRadius is assigned to the radius, the Point part of Circle is constructed thru a name to Point’s constructor inside the initializer listing. This list is the whole thing among the: and the below.
Circle::Circle(int atx,int aty,int theRadius) : Point(atx,aty)
Incidentally, constructor type initialization can be used for all built-in types.
int a1(10) ;
int a2=10 ;
What Is Polymorphism?
Polymorphism is a regular term which means “many shapes”. In C++ the only form of Polymorphism is overloading of functions. For instance, several capabilities called SortArray( arraytype ) in which sortarray might be an array of ints or doubles.
We’re best inquisitive about the OOP form of polymorphism right here, although. This is finished by making a characteristic (e.G. Draw() ) virtual inside the base class Point and then overriding it in the derived magnificence Circle.
Polymorphism?
Although the characteristic Draw() is virtual in the derived magnificence Circle, this is not certainly wished—it’s just a reminder to me that this it’s miles digital. If the feature in a derived class fits a digital characteristic within the base magnificence on name and parameter types, it’s miles mechanically digital.
Drawing a point and drawing a circle are very exclusive operations with best the coordinates of the factor and circle in common, so it’s important that the proper Draw() is referred to as. How the compiler manages to generate code that receives the proper virtual characteristic will be protected in a destiny tutorial.
C++ Constructors
Constructors
A constructor is a function that initializes the participants of an item. A constructor handiest knows how to build an object of its very own magnificence.
Constructors are not mechanically inherited between the base and derived instructions. If you don’t deliver one in the derived magnificence, a default may be supplied but this will now not do what you need.
If no constructor is provided then a default one is created by the compiler with none parameters. There need to usually be a constructor, despite the fact that it is the default and empty. If you supply a constructor with parameters then a default will NOT be created.
Some points approximately constructors:
- simply capabilities with the identical name because the class.
- supposed to initialize the contributors of the magnificence whilst an instance of that magnificence is created.
- immediately (except thru initializer lists)
- Constructors are never virtual.
- Multiple constructors for the identical magnificence may be defined. They have to have specific parameters to differentiate them.
There is lots more to study constructors, for example, default constructors, challenge, and replica constructors. These could be mentioned within the next lesson.
Tidying Up C++ Destructors
A destructor is a class member function that has the same name as the constructor (and the class ) but with a ~ (tilde) in front.
~Circle() ;
When an object is going out of scope or extra rarely is explicitly destroyed, its destructor is called. For instance, if the object has dynamic variables which includes pointers, then those need to be freed and the destructor is the correct region.
C++ Destructors
Unlike constructors, destructors can and ought to be made virtual if you have derived classes. In the Point and Circle instructions instance, the destructor isn’t needed as there is no cleanup work to be executed (it simply serves as an example). Had there been dynamic member variables (like tips) then the ones could have required liberating to save you reminiscence leaks.
Also, when the derived magnificence adds participants that require tidying up, virtual destructors are wished. When virtual, the maximum derived class destructor is referred to as first, then its on the spot ancestor’s destructor is known as, and so on as much as the base magnificence.
In our instance,
~Circle() ;
then
~Point() ;
The base classes destructor is referred to as closing.
This completes this lesson. In the next lesson, find out about default constructors, replica constructors, and assignment.