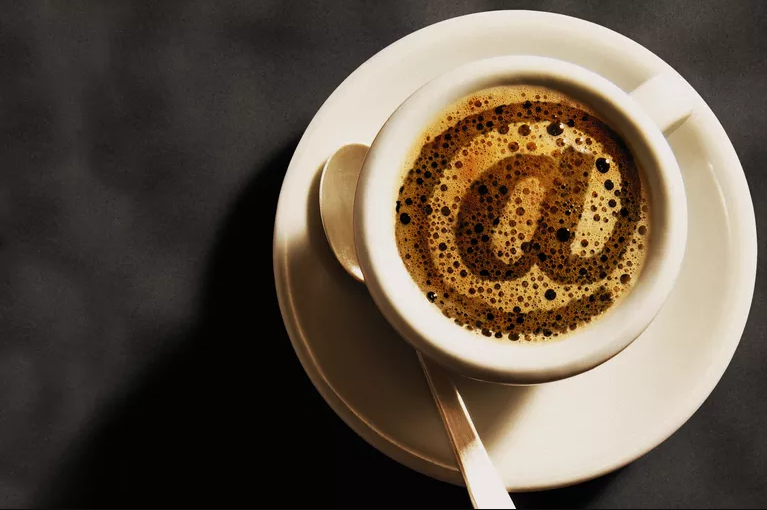
Instance variables, begin with an at signal (@) and can be referenced best inside magnificence techniques. They range from neighborhood variables in that they don’t exist inside any specific scope. Instead, a comparable variable desk is stored for each instance of a class. Instance variables live inside a category example, so so long as that instance remains alive, so will the instance variables.
Instance variables can be referenced in any technique of that magnificence. All techniques of a class use the identical example variable table, as opposed to neighborhood variables in which every method may have a extraordinary variable table. It is possible to access instance variables with out first defining them, however. This will not increase an exception, but the variable’s cost will be nil and a caution can be issued in case you’ve run Ruby with the -w switch.
Instance Variables, in Ruby Variables
This instance demonstrates using instance variables. Note that the shebang contains the -w switch, so that it will print warnings ought to they occur. Also, note the wrong utilization outside of a method in the elegance scope. This is inaccurate and discussed beneath.
#!/usr/bin/env ruby -w class TestClass # Incorrect! @test = “monkey” def initialize @value = 1337 end def print_value # OK puts @value end def uninitialized # Technically OK, generates warning puts @monkey end end t = TestClass.new t.print_value t.uninitialized
Why is the @check variable wrong? This has to do with scope and the way Ruby implements things. Within a technique, the example variable scope refers back to the specific example of that elegance.
However, in the magnificence scope (within the class, but outside of any techniques), the scope is the magnificence example scope. Ruby implements the class hierarchy by using instantiating Class gadgets, so there is a 2nd instance at play right here.
The first example is an example of the Class elegance, and that is in which @check will cross. The 2d instance is the instantiation of TestClass, and that is in which @cost will cross. This receives a chunk confusing, but simply don’t forget to in no way use @instance_variables out of doors of strategies. If you want magnificence-huge storage, use @@class_variables, which can be used everywhere inside the magnificence scope (inside or outside of strategies) and will behave the identical.
Accessors
You usually can not get entry to instance variables from out of doors of an object. For example, inside the above instance, you can’t in reality name t.Cost or t.@value to get right of entry to the example variable @fee. This could smash the guidelines of encapsulation. This also applies to instances of baby training, they can not get admission to instance variables belonging to the discern class despite the fact that they are technically the same type. So, that allows you to provide access to example variables, accessor techniques have to be declared.
The following instance demonstrates how accessor techniques can be written. However, notice that Ruby gives a shortcut and that this situation simplest exists to reveal you how the accessor methods work. It’s normally not commonplace to peer accessor methods written in this way unless some form of extra common sense is wanted for the accessor.
#!/usr/bin/env ruby class Student def initialize(name,age) @name, @age = name, age end # Name reader, assume name can’t change def name @name end # Age reader and writer def age @age end def age=(age) @age = age end end alice = Student.new(“Alice”, 17) # It’s Alice’s birthday alice.age += 1 puts “Happy birthday #{alice.name}, \ you’re now #{alice.age} years old!”
Instance Variables in Ruby Variables
The shortcuts make things a piece less complicated and extra compact. There are 3 of these helper methods. They ought to be run inside the class scope (within the elegance however outside of any strategies), and will dynamically outline strategies similar to the strategies described inside the above example. There’s no magic happening here, and that they appear like language key phrases, however they absolutely are just dynamically defining strategies. Also, these accessors generally pass on the top of the class. That gives the reader an instant evaluation of which member variables might be to be had outdoor the class or to toddler lessons.
There are 3 of those accessor techniques. They every take a listing of symbols describing the instance variables to be accessed.
- Attr_reader – Define “reader” techniques, including the call approach inside the above instance.
- Attr_writer – Define “creator” methods together with the age= method in the above example.
- Attr_accessor – Define each “reader” and “creator” techniques.
!/usr/bin/env ruby class Student attr_reader :call attr_accessor :age def initialize(call,age) @call, @age = call, age cease give up alice = Student.New(“Alice”, 17)
It’s Alice’s birthday alice.Age += 1 places “Happy birthday #alice.Call, you are now #alice.Age years old!”
When to apply Instance Variables
Now that you understand what instance variables are, while do you use them? Instance variables should be used when they represent the kingdom of the object. A scholar’s call and age, their grades, and so on. They should not be used for temporary garage, that is what nearby variables are for. However, they could possibly be used for transient storage among approach calls for multi-stage computations. However if you’re doing this, you may want to reconsider your method composition and make these variables into approach parameters as a substitute.