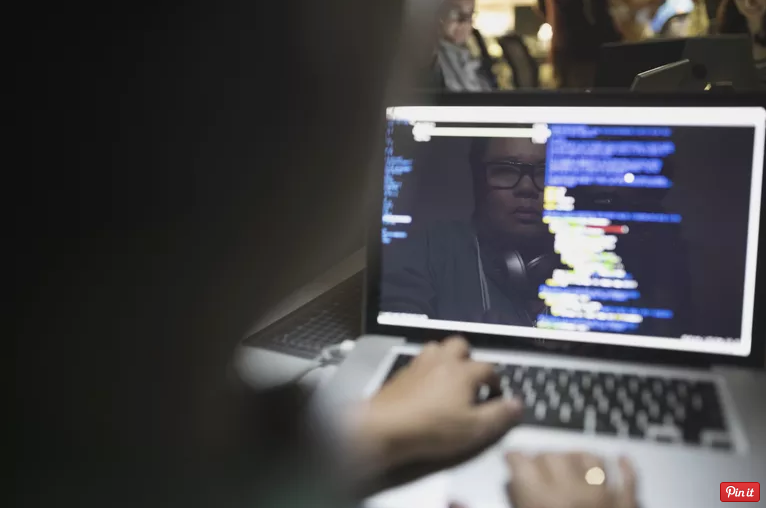
GDI+ Graphics, GDI+ is the way to attract shapes, fonts, pictures or usually something photo in Visual Basic .NET.
This article is the first a part of a entire advent to using GDI+ in Visual Basic .NET.
GDI+ is an uncommon part of .NET. It turned into right here before .NET (GDI+ changed into released with Windows XP) and it doesn’t percentage the same replace cycles because the .NET Framework. Microsoft’s documentation normally states that Microsoft Windows GDI+ is an API for C/C++ programmers into the Windows OS. But GDI+ additionally includes the namespaces used in VB.NET for software program-based totally photos programming.
GDI+ Graphics, WPF
But it’s not the most effective pix software program provided through Microsoft, mainly considering Framework 3.0. When Vista and 3.0 have been delivered, the absolutely new WPF became delivered with it. WPF is a excessive-degree, hardware improved method to images. As Tim Cahill, Microsoft WPF software program team member, puts it, with WPF “you describe your scene using high-stage constructs, and we’ll worry approximately the rest.” And the truth that it’s hardware accelerated method which you do not have to drag down the operation of your PC processor drawing shapes at the display. Much of the actual paintings is completed via your pictures card.
We’ve been right here before, but. Every “first-rate jump ahead” is usually observed by way of a few stumbles backward, and besides, it will take years for WPF to work its way thru the zillions of bytes of GDI+ code. That’s specifically authentic considering the fact that WPF just about assumes which you’re working with a excessive-powered machine with masses of memory and a hot pix card. That’s why many PCs could not run Vista (or at the least, use the Vista “Aero” snap shots) when it was first introduced. So this series is still available at the website online for any and all who continue to want to use it.
GDI+ Graphics, Good Ol’ Code
GDI+ isn’t always some thing that you may drag onto a shape like different components in VB.NET. Instead, GDI+ objects typically need to be brought the vintage way — via coding them from scratch! (Although, VB .NET does encompass some of very accessible code snippets that may absolutely help you.)
To code GDI+, you use items and their members from some of .NET namespaces. (At the prevailing time, these are simply simply wrapper code for Windows OS objects which virtually do the paintings.)
GDI+ Graphics, Namespaces
The namespaces in GDI+ are:
System.Drawing
This is the core GDI+ namespace. It defines gadgets for fundamental rendering (fonts, pens, fundamental brushes, and many others.) and the most vital object: Graphics. We’ll see extra of this in only some paragraphs.
System.Drawing.Drawing2D
This gives you items for extra advanced -dimensional vector snap shots. Some of them are gradient brushes, pen caps, and geometric transforms.
System.Drawing.Imaging
If you want to exchange graphical images – this is, alternate the palette, extract image metadata, control metafiles, and so forth – this is the one you want.
System.Drawing.Printing
To render photos to the printed web page, interact with the printer itself, and layout the overall appearance of a print activity, use the items right here.
System.Drawing.Text
You can use collections of fonts with this namespace.
Graphics Object
The location to begin with GDI+ is the Graphics object. Although the stuff you draw show up on your reveal or a printer, the Graphics item is the “canvas” that you draw on.
But the Graphics item is likewise one of the first resources of misunderstanding while the usage of GDI+. The Graphics item is continually related to a selected tool context. So the first hassle that truly each new scholar of GDI+ confronts is, “How do I get a Graphics item?”
There are essentially two approaches:
1.You can use the e occasion parameter that is handed to the OnPaint event with the PaintEventArgs item. Several activities skip the PaintEventArgs and you can use the to consult the Graphics object this is already being used by the tool context.
2.You can use the CreateGraphics technique for a device context to create a Graphics object. Here’s an instance of the primary method:
Protected Overrides Sub OnPaint( _
ByVal e As System.Windows.Forms.PaintEventArgs)
Dim g As Graphics = e.Graphics
g.DrawString("About Visual Basic" & vbCrLf _
& "and GDI+" & vbCrLf & "A Great Team", _
New Font("Times New Roman", 20), _
Brushes.Firebrick, 0, 0)
MyBase.OnPaint(e)
End Sub
Click Here to display the example
Add this into the Form1 magnificence for a standard Windows Application to code it your self.
In this situation, a Graphics object is already created for the shape Form1. All your code has to do is create a nearby example of that object and use it to attract on the equal shape. Notice that your code Overrides the OnPaint method. That’s why MyBase.OnPaint(e) is executed at the quit. You want to make certain that if the base object (the one you are overriding) is doing something else, it receives a hazard to do it. Often, your code works without this, but it is a good idea.
PaintEventArgs
You also can get a Graphics object using the PaintEventArgs item surpassed for your code within the OnPaint and OnPaintBackground methods of a Form. The PrintPageEventArgs exceeded in a PrintPage event will contain a Graphics item for printing. It’s even viable to get a Graphics item for some snap shots. This can will let you paint right at the image the identical way you would paint on a Form or element.
Event Handler
Another variant of approach one is to feature an event handler for the Paint event for the form. Here’s what that code looks as if:
Private Sub Form1_Paint( _
ByVal sender As Object, _
ByVal e As System.Windows.Forms.PaintEventArgs) _
Handles Me.Paint
Dim g As Graphics = e.Graphics
g.DrawString("About Visual Basic" & vbCrLf _
& "and GDI+" & vbCrLf & "A Great Team", _
New Font("Times New Roman", 20), _
Brushes.Firebrick, 0, 0)
End Sub
CreateGraphics
The second technique to get a Graphics item to your code uses a CreateGraphics method that is to be had with many additives. The code looks like this:
Private Sub Button1_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) _
Handles Button1.Click
Dim g = Me.CreateGraphics
g.DrawString("About Visual Basic" & vbCrLf _
& "and GDI+" & vbCrLf & "A Great Team", _
New Font("Times New Roman", 20), _
Brushes.Firebrick, 0, 0)
End Sub
There are more than one variations right here. This is within the Button1.Click occasion because while Form1 repaints itself within the Load event, our pix are lost. So we must upload them in a later occasion. If you code this, you may notice that the snap shots are misplaced when Form1 needs to be redrawn. (Mimimize and maximize again to peer this.) That’s a huge advantage to the use of the primary technique.
Most references advocate the use of the primary technique for the reason that your portraits will be repainted robotically. GDI+ can be complex!