Using NetBeans and Swing
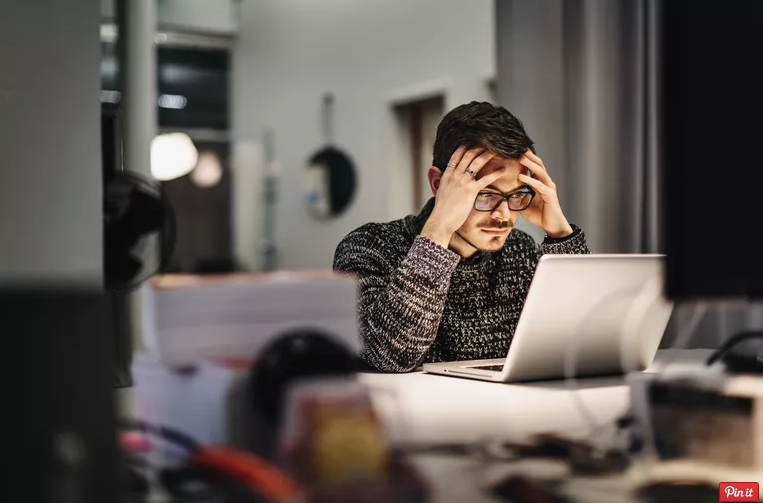
Coding,A graphical person interface (GUI) built using the Java NetBeans platform is made from numerous layers of boxes. The first layer is the window used to transport the application across the display screen of your computer. This is known as the pinnacle-degree box, and its task is to offer all other packing containers and graphical additives an area to work in. Typically for a computer software, this top-level container could be made using the
JFrame
class.
You can add any number of layers in your GUI design, relying on its complexity. You can region graphical components (e.G., text bins, labels, buttons) at once into the
JFrame
, or you may group them in different boxes.
The layers of the GUI are called the containment hierarchy and can be idea of as a own family tree. If the
JFrame
Is the grandfather sitting at the pinnacle, then the subsequent box can be concept of as the daddy and the additives it holds because the children.
For this example, we will build a GUI with a
JFrame
containing two
JPanels
and a
JButton
The first
JPanel
will hold a
JLabel
and
JComboBox
The second
JPanel
will hold a
JLabel
and a
JList
Only one
JPanel
(and therefore the graphical additives it includes) will be visible at a time. The button will be used to exchange the visibility of the two
JPanels
There are two methods to construct this GUI using NetBeans. The first is to manually kind in the Java code that represents the GUI, which is mentioned in this article. The second is to use the NetBeans GUI Builder tool for building Swing GUIs.
For facts on using JavaFX as opposed to Swing to create a GUI, see What is JavaFX?
Note: The entire code for this assignment is at Example Java Code for Building A Simple GUI Application.
Coding,Setting Up the NetBeans Project
Create a new Java Application venture in NetBeans with a major elegance We’ll name the project
GuiApp1
Check Point: In the Projects window of NetBeans need to be a pinnacle-degree GuiApp1 folder (if the call isn’t in ambitious, proper-click on the folder and choose
Set as Main Project
). Beneath the
GuiApp1
Older should be a Source Packages folder with a applications folder referred to as GuiApp1. This folder contains the primary magnificence called
GuiApp1
.java.
Before we upload any Java code, add the subsequent imports to the pinnacle of the
GuiApp1
class, between the
package GuiApp1
line and the
public class GuiApp1
:
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JComboBox;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JList;
import java.awt.BorderLayout;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
These imports mean that each one the classes we need to make this GUI software can be to be had for us to use.
Within the primary technique, upload this line of code:
public static void main(String[] args) { // existing main method
new GuiApp1(); // add this line
This way that the primary thing to do is to create a brand new
GuiApp1
Item. It’s a pleasing brief-reduce for example programs, as we simplest want one elegance. For this to paintings, we need a constructor for the
GuiApp1
class, so add a new method:
public GuiApp1
{
}
In this approach, we will placed all the Java code had to create the GUI, that means that each line any more may be in the
GuiApp1()
method.
Coding,Building the Application Window Using a JFrame
Design Note: You would possibly have seen Java code published that indicates the class (i.E.,
GuiApp1
) extended from a
JFrame
. This magnificence is then used as the principle GUI window for an software. There simply isn’t always any need to do that for a normal GUI utility. The best time you’ll need to extend the
JFrame
Magnificence is if you need to make a more specific sort of
JFrame
(take a look at What is Inheritance? For extra records on making a subclass).
As noted earlier, the first layer of the GUI is an utility window made from a
JFrame
To create a
JFrame
object, call the
JFrame
constructor:
JFrame guiFrame = new JFrame();
Next, we’ll set the conduct of our GUI application window, using those four steps:
- Ensure that the utility closes when the person closes the window so that it does no longer maintain to run unknown within the background:
guiFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
2. Set a identify for the window so the window does not have a clean name bar. Add this line:
guiFrame.setTitle(“Example GUI”);
3. Set the window length, so that the window is sized to accommodate the graphical components you area into it.
guiFrame.setSize(300,250);
Design Note: An opportunity option for setting the dimensions of the window is to call the
pack()
method of the
JFrame
Elegance. This method calculates the scale of the window primarily based at the graphical additives it carries. Because this pattern application would not need to trade its window length, we’re going to simply use the
setSize()
method.
4. Center the window to appear inside the middle of the computer display so that it does no longer seem inside the pinnacle left-hand nook of the display screen:
guiFrame.setLocationRelativeTo(null);
Coding,Adding the Two JPanels
The two lines here create values for the
JComboBox
and
JList
objects we’ll be creating shortly, using two
String
Arrays. This makes it simpler to populate some example entries for those additives:
String[] fruitOptions = {“Apple”, “Apricot”, “Banana”
,”Cherry”, “Date”, “Kiwi”, “Orange”, “Pear”, “Strawberry”};
String[] vegOptions = {“Asparagus”, “Beans”, “Broccoli”, “Cabbage”
, “Carrot”, “Celery”, “Cucumber”, “Leek”, “Mushroom”
, “Pepper”, “Radish”, “Shallot”, “Spinach”, “Swede”
, “Turnip”};
Create the first JPanel Object
Now, let’s create the first
JPanel
object. It will contain a
JLabel
and a
JComboBox
. All three are created via their constructor methods:
final JPanel comboPanel = new JPanel();
JLabel comboLbl = new JLabel(“Fruits:”);
JComboBox fruits = new JComboBox(fruitOptions);
Notes on the above three lines:
The
JPanel
Variable is said very last. This method that the variable can simplest maintain the
JPanel
It truly is created on this line. The end result is that we will use the variable in an inner magnificence. It will become apparent why we need to afterward within the code.
The
JLabel
and
JComboBox
Have values passed to them to set their graphical properties. The label will seem as “Fruits:” and the combobox will now have the values contained inside the
fruitOptions
array declared earlier.
The
add()
method of the
JPanel
places graphical components into it. A
JPanel
Makes use of the FlowLayout as its default format supervisor. This is pleasant for this utility as we need the label to sit down subsequent to the combobox. As long as we upload the
JLabel
first, it will look fine:
comboPanel.add(comboLbl);
comboPanel.add(fruits);
Create the Second JPanel Object
The second
JPanel
follows the same pattern. We’ll add a
JLabel
and a
JList
And set the values of those components to be “Vegetables:” and the second one
String
array
vegOptions
. The only other difference is the use of the
setVisible()
method to hide the
JPanel
. Don’t forget there will be a
JButton
controlling the visibility of the two
JPanels
. For this to paintings, one desires to be invisible on the start. Add these lines to set up the second
JPanel
:
final JPanel listPanel = new JPanel();
listPanel.setVisible(false);
JLabel listLbl = new JLabel(“Vegetables:”);
vegs.setLayoutOrientation(JList.HORIZONTAL_WRAP);
listPanel.add(listLbl);
listPanel.add(vegs);
One line worth noting within the above code is using the
setLayoutOrientation()
method of the
JList
. The
HORIZONTAL_WRAP