Cloud Firestore + Android is easy A even as in the past, Google has launched Cloud Firestore. Cloud Firestore is a cloud-primarily based NoSQL database, which Google positions as a substitute for the Realtime Database. In this article I want to tell how to start using it.
Cloud Firestore + Android is easy Opportunities
Cloud Firestore + Android is easy Cloud Firestore allows you to keep information on a remote server, effortlessly get get admission to to them and screen changes in real time. The documentation has an super assessment of Cloud Firestore and Realtime Database.
Cloud Firestore + Android is easyCreation and connection to the project
Cloud Firestore + Android is easy In the Firebase console, select Database and click on Create database. Next, choose the get right of entry to settings. It’s sufficient take a look at mode for acquaintance, however at the manufacturing it is higher to approach this issue extra critically. You can examine more about get entry to modes here.
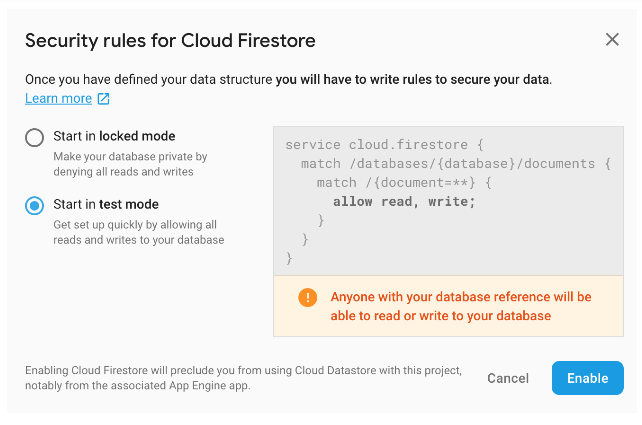
To configure the project, we ought to make the following steps:
01.Add Firebase to the task consistent with the commands from right here. 02.Add dependency to app/construct.Gradle
implementation ‘com.google.firebase:firebase-firestore:18.1.0’
Now the entirety is ready.
To get familiar with the fundamental techniques of working with Cloud Firestore, I made a simple software. The supply code for the app can be determined here:
To make the utility work you want to create a undertaking in the Firebase console and add the google-services.Json document to the project in Android Studio.
Data storage structure
Firestore makes use of collections and documents to save records. A record is an entry that contains any fields. Documents are blended into collections. Also, the document may incorporate nested collections, but on Android it isn’t always supported. If we draw an analogy with the SQL database, the collection is a table, and the file is an access in this table. One series may additionally contain files with a one of a kind set of fields.
Receive and write data
In order to get all of the documents of a collection, the following code is enough
remoteDB.collection(“Tasks”)
.get()
.addOnSuccessListener { querySnapshot ->
// Successfully received data. List in querySnapshot.documents
}
.addOnFailureListener { exception ->
// An error occurred while getting data
}
Here we request all documents from the Tasks series.
The library permits you to create queries with parameters. The following code suggests the way to get documents from the gathering by circumstance
remoteDB.collection(“Tasks”)
.whereEqualTo("title", "Task1")
.get()
.addOnSuccessListener { querySnapshot ->
// Successfully received data. List in querySnapshot.documents
}
.addOnFailureListener { exception ->
// An error occurred while getting data
}
Here we request all documents from the Tasks collection, wherein the name discipline corresponds to the price of Task1.
While getting the files, they can be right now converted into our statistics lessons
remoteDB.collection(“Tasks”)
.get()
.addOnSuccessListener { querySnapshot ->
// Successfully received data. List in querySnapshot.documents
val taskList: List<RemoteTask> = querySnapshot.toObjects(RemoteTask::class.java)
}
.addOnFailureListener { exception ->
// An error occurred while getting data
}
For writing, you have to create a Hashmap with facts (in which the call of the sphere acts as a key, and the cost of this field as a fee) and switch it to the library. You can see that within the following code
val taskData = HashMap<String, Any>()
taskData["title"] = task.title
taskData["created"] = Timestamp(task.created.time / 1000, 0)
remoteDB.collection("Tasks")
.add(taskData)
.addOnSuccessListener {
// Successful write
}
.addOnFailureListener {
// There was an error while writing
}
In this situation, a new document might be created and the Firestore will generate an identity for it. To set your very own id you need to do the subsequent
val taskData = HashMap<String, Any>()
taskData["title"] = task.title
taskData["created"] = Timestamp(task.created.time / 1000, 0)
remoteDB.collection("Tasks")
.document("New task")
.set(taskData)
.addOnSuccessListener {
// Successful write
}
.addOnFailureListener {
// There was an error while writing
}
In this case, if there is no record with identification equal to New task, then it’ll be created, and if it’s miles, then the desired fields could be updated.
Another option to create/replace a record
remoteDB.collection("Tasks")
.document("New task")
.set(mapToRemoteTask(task))
.addOnSuccessListener {
// Successful write
}
.addOnFailureListener {
// There was an error while writing
}
Subscribe to changes
Firestore allows you to enroll in facts adjustments. You can join modifications inside the series as well as modifications to a specific record
remoteDB.collection("Tasks")
.addSnapshotListener { querySnapshot, error ->
// querySnapshot - list of changes
// error - error
}
QuerySnapshot.Files — consists of an updated listing of all documents querySnapshot.DocumentChanges — consists of a list of modifications. Each object consists of a changed file and a form of trade. Three forms of changes are possible: ADDED — files brought MODIFIED — files up to date, REMOVED — documents deleted
Loading large amounts of data
Realtime Database provides more or less convenient mechanism for loading huge amounts of facts, which primarily based on manually editing a json record and loading it. Firestore does not provide some thing. It became very inconvenient to add new documents till I found a way to down load a large amount of records as effortlessly as feasible. So which you do now not have such issues as me, I will connect the instructions beneath on how to fast and easily load a huge quantity of statistics. The education become located at the Internet.
01.Install Node.Js and npm 02.Install the firebase-admin package deal by means of jogging the command
npm install firebase-admin — save
3.Generate a json document with series statistics. An example can be located inside the Tasks.Json file.
4.For loading we want an get entry to key. How to get it is properly described in this article.
5.In the export.Js record fill together with your statistics require(‘./firestore_key.Json’) — document with get right of entry to key. I put it inside the folder with the script — the name of your firestore database “./json/Tasks.Json” — the path to the record wherein the information lie [‘created’] — listing of discipline names with type Timestamp
6.Run script
node export.js
Summary
I used Cloud Firestore in certainly one of my tasks and did not have any severe troubles. One of my collections includes about 15,000 documents and requests for it bypass fairly quickly and this is with out the use of indexes. Using Cloud Firestore together with Room and Remote Config, you could significantly reduce the quantity of calls to the database and now not exceed the free limits. By using a unfastened charge you can study 50,000 documents, write 20,000 and remove 20,000 in line with a day.
Example of running utility
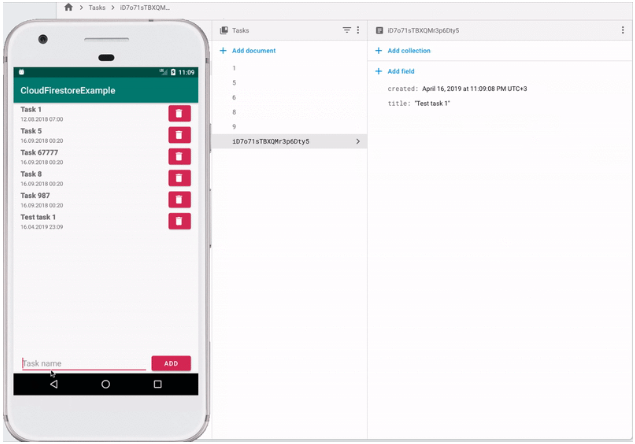