Slide Up / Down Animations,In android, Slide Up and Slide Down animations are used to alternate the arrival and behavior of the items over a specific c program languageperiod of time. The Slide Up and Slide Down animations will provide a higher appearance and feel for our packages.
Because, the animations are beneficial while we need to notify users approximately the alternate’s taking place in our app, inclusive of new content loaded or new moves available, and so on.
To create an animation effect to the gadgets in our android utility, we need to observe beneath steps.
Slide Up / Down Animations,Create XML File to Define Animation
We want to create an xml report that defines the kind of animation to perform in a brand new folder anim beneath res directory (res à anim à slide_up.Xml) with required properties.
To use Slide Up or Slide Down animations in our android programs, we want to define a new xml document with tag like as shown beneath.
For Slide Up animation, we need to set android:fromYScale=”1.Zero” and android:toYScale=”0.Zero” like as proven under.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:toXScale="1.0"
android:toYScale="0.0" />
</set>
For Slide Down animation, we need to set android:fromYScale=”0.0″ and android:toYScale=”1.0″ like as shown below.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="0.0"
android:toXScale="1.0"
android:toYScale="1.0" />
</set>
Although, once we are performed with creation of required animation XML documents, we need to load those animation files the use of distinct properties.
Slide Up / Down Animations,Android Load and Start the Animation
we can perform animations with the aid of the usage of AnimationUtils factor strategies together with loadAnimation().
Though ,following is the code snippet of loading and beginning an animation the usage of loadAnimation() and startAnimation() methods.
ImageView img = (ImageView)findViewById(R.id.imgvw);
Animation aniSlide = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.slide_up);
img.startAnimation(aniSlide);
If you examine above code snippet, we’re adding an animation to the photo using loadAnimation() technique. The 2d parameter in loadAnimation() method is the call of our animation xml document.
Here we used some other technique startAnimation() to use the defined animation to imageview item.
Now we can see the way to enforce slide up and slide down animations for imageview on button click on in android applications with examples.
Slide Up / Down Animations, Example
Although, following is the instance of implementing a slide up and slide down animations to slip an photo up or down on button click in android packages.
Create a brand new android software the use of android studio and deliver names as SlideUpDownExample.
In case if you are not privy to creating an app in android studio check this text Android Hello World App.
Though Once we create an utility, open activity_main.Xml report from reslayout folder route and write the code like as shown under.
Slide Up / Down Animations,activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="10dp"
android:paddingRight="10dp">
<ImageView android:id="@+id/imgvw"
android:layout_width="wrap_content"
android:layout_height="250dp"
android:src="@drawable/bangkok"/>
<Button
android:id="@+id/btnSlideDown"
android:layout_below="@+id/imgvw"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Slide Down" android:layout_marginLeft="100dp" />
<Button
android:id="@+id/btnSlideUp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/btnSlideDown"
android:layout_toRightOf="@+id/btnSlideDown"
android:text="Slide Up" />
</RelativeLayout>
As discussed, we want to create an xml documents to define slide up and slide down animations in new folder anim below res directory (res à anim à slide_up.Xml, slide_down.Xml) with required houses.
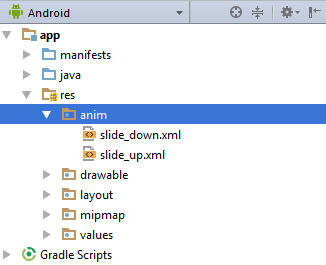
Now open slide_up.xml file and write the code to set slide up animation properties like as shown below.
slide_up.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:toXScale="1.0"
android:toYScale="0.0" />
</set>
Now open slide_down.xml file and write the code to set slide down animation properties like as shown below.
slide_down.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="0.0"
android:toXScale="1.0"
android:toYScale="1.0" />
</set>
Now open your main activity file MainActivity.java from \java\com.tutlane.slideupdownexample path and write the code like as shown below.
MainActivity.java
package com.tutlane.slideupdownexample;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
private Button btnSDown;
private Button btnSUp;
private ImageView img;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnSDown = (Button)findViewById(R.id.btnSlideDown);
btnSUp = (Button)findViewById(R.id.btnSlideUp);
img = (ImageView)findViewById(R.id.imgvw);
btnSDown.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Animation animSlideDown = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.slide_down);
img.startAnimation(animSlideDown);
}
});
btnSUp.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Animation animSlideUp = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.slide_up);
img.startAnimation(animSlideUp);
}
});
}
}
If you look at above code, we are including an animation to the photograph the use of loadAnimation() technique used startAnimation() technique to apply the defined animation to imageview object.
Output of Android Slide Up / Down Animation Example
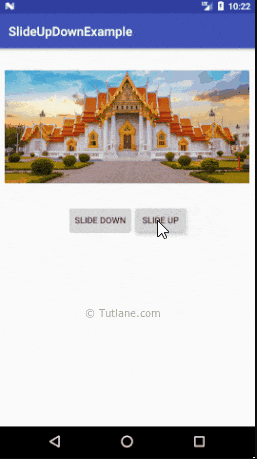
Because, If you have a look at above end result, whenever we are clicking on Slide Up or Slide Down buttons, the photograph length varies primarily based on our functionality.
This is how we can put in force slide up and slide down animations for imageview in android applications primarily based on our requirements.