In android, Menu is part of consumer interface (UI) element that is used to deal with a few commonplace functionality across the application. By the usage of Menus in our programs, we can offer better and steady person enjoy at some point of the software.
We can use Menu APIs to represent consumer actions and different alternatives in our android utility activities.
Following is the pictorial illustration of the usage of menus in android utility.
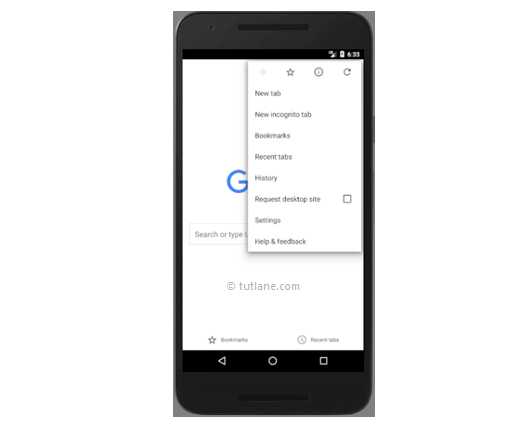
In android, we can outline a Menu in separate XML record and use that file in our activities or fragments primarily based on our requirements.
Define a Android Menu in XML File
For all menu kinds, Android affords a fashionable XML format to outline menu gadgets. Instead of constructing a menu in our hobby’s code, we should outline a menu and all its objects in an XML menu useful resource and cargo menu resource as a Menu object in our interest or fragment.
In android, to outline menu, we want to create a brand new folder menu inner of our undertaking useful resource listing (res/menu/) and upload a new XML file to construct the menu with the following elements.
Following is the instance of defining a menu in XML document (menu_example.Xml).
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/mail"
android:icon="@drawable/ic_mail"
android:title="@string/mail" />
<item android:id="@+id/upload"
android:icon="@drawable/ic_upload"
android:title="@string/upload"
android:showAsAction="ifRoom" />
<item android:id="@+id/share"
android:icon="@drawable/ic_share"
android:title="@string/share" />
</menu>
The element in menu helps extraordinary form of attributes to define object’s behaviour and appearance. Following are the some of typically used attributes in android packages.
Attribute | Description |
android:id | It is used to uniquely identify element in application. |
android:icon | It is used to set the item’s icon from drawable folder. |
android:title | It is used to set the item’s title |
android:showAsAction | It is used to specify how the item should appear as an action item in the app bar. |
In case if we need to add submenu in menu item, then we want to feature a detail as the child of an . Following is the instance of defining a submenu in menu object.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/file"
android:title="@string/file" >
<!-- "file" submenu -->
<menu>
<item android:id="@+id/create_new"
android:title="@string/create_new" />
<item android:id="@+id/open"
android:title="@string/open" />
</menu>
</item>
</menu>
Load Android Menu from an Activity
Once we are done with creation of menu, we need to load the menu resource from our activity using MenuInflater.inflate() like as shown below.
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.menu_example, menu);
}
If you have a look at above code we are calling our menu using MenuInflater.Inflate() technique within the shape of R.Menu.Menu_file_name. Here our xml record call is menu_example.Xml so we used file call menu_example.
Handle Android Menu Click Events
In android, we will manage a menu object click occasions the use of ItemSelected() occasion based at the menu kind. Following is the instance of coping with a context menu item click event the use of onContextItemSelected().
@Override
public boolean onContextItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.mail:
// do something
return true;
case R.id.share:
// do something
return true;
default:
return super.onContextItemSelected(item);
}
}
If you take a look at above code, the getItemId() approach will get the identity of selected menu item primarily based on that we can carry out our moves.
Android Different Types of Menus
In android, we’ve a three fundamental type of Menus to be had to define a fixed of options and movements in our android packages.
Following are the typically used Menus in android applications. Options Menu Context Menu Popup Menu
Android Options Menu
In android, Options Menu is a primary collection of menu items for an activity and it’s miles beneficial to enforce movements which have a worldwide impact on the app, including Settings, Search, and so forth.
To recognize more about Options Menu, test this Android Options Menu with Examples.
Android Context Menu
In android, Context Menu is a floating menu that appears when the user plays an extended click on an detail and it is beneficial to put into effect an movements that impact the selected content or context frame.
To recognise greater about Context Menu, check this Android Context Menu with Examples.
Android Popup Menu
In android, Popup Menu shows a list of items in a vertical list that’s anchored to the view that invoked the menu and it’s beneficial for presenting an overflow of moves that related to particular content material.
To know extra approximately Popup Menu, test this Android Popup Menu with Examples.