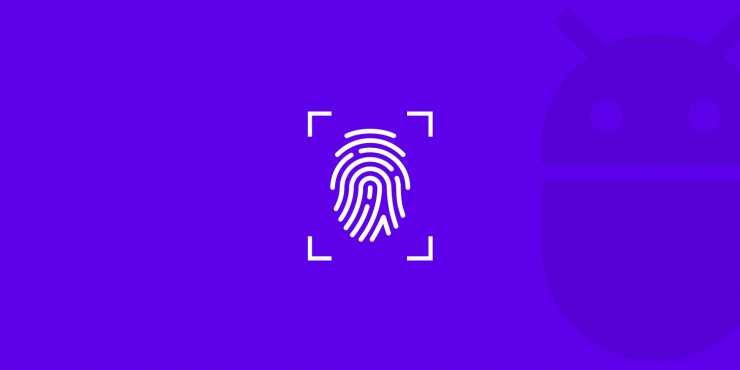
Integrating Fingerprint authentication, has been a massive soar towards a better consumer revel in in smartphones. Having this golden characteristic on your consumer’s telephone.
And looking to integrate this selection on your programs to growth protection and the bonus of better person experience is worth a shot. Let’s see how we are able to speedy integrate a simple FingerPrint authentication into an android app.
While integrating Fingerprint auth in our programs, we want to take care of pretty plenty of factors concerning the hardware of the tool our users are using.
We recognise that best devices walking android Marshmallow and above have guide for Fingerprint scanners. Additionally to apply the Android’s integrated Fingerprint functions the person desires to have enabled fingerprint security in the tool and have as a minimum one fingerprint registered in the device.
Let’s preserve these points in thoughts and we want to test this stuff earlier than dispatching a listener for fingerprint authentication.
Integrating Fingerprint authentication, Permission for Fingerprint use
We want our utility’s manifest record to describe that our application needs permission to apply Fingerprint. Let’s add the following line within the appear tag in the AndroidManifest.Xml file.
Integrating Fingerprint authentication, Checking Fingerprint availability
As I referred to above, we want to verify that the consumer runs suitable Android version, the device has the essential hardware for the Fingerprint scanner and the person has as a minimum one fingerprint already registered in the tool.
Integrating Fingerprint authentication, Write down the following method on your pastime’s magnificence or create a separate java document and drop this method in there.
public boolean checkFingerprintSettings(Context context) { final String TAG = “FP-Check”; //Check for android version, FingerPrint is not available below Marshmallow if (Build.VERSION.SDK_INT < Build.VERSION_CODES.M) { Log.d(TAG, “This Android version does not support fingerprint authentication.”); return false; } //Check whether the security option for phone is set. //ie. LockScreen is enabled or not. KeyguardManager keyguardManager = (KeyguardManager) context.getSystemService(KEYGUARD_SERVICE); FingerprintManager fingerprintManager = (FingerprintManager) context.getSystemService(FINGERPRINT_SERVICE); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) { if (!keyguardManager.isKeyguardSecure()) { Log.d(TAG, “User hasn’t enabled Lock Screen”); return false; } } //check if user have registered any fingerprints if (!fingerprintManager.hasEnrolledFingerprints()) { Log.d(TAG, “User hasn’t registered any fingerprints”); return false; } //check for app permissions //Make sure you have mentioned the permission on AndroidManifest.xml if (ActivityCompat.checkSelfPermission(context, Manifest.permission.USE_FINGERPRINT) != PackageManager.PERMISSION_GRANTED) { Log.d(TAG, “User hasn’t granted permission to use Fingerprint”); return false; } Log.d(TAG, “Fingerprint authentication is set.”); return true; }
Creating a Fingerprint Helper class
Next, we want to create a helper elegance by way of extending the FingerprintManager.AuthenticationCallback and we’ll create an interface inner this magnificence for the authentication listner.
@RequiresApi(api = Build.VERSION_CODES.M) public class FingerprintHelper extends AuthenticationCallback{ private FingerprintHelperListener listener; public FingerprintHelper(FingerprintHelperListener listener) { this.listener = listener; } //interface for the listner interface FingerprintHelperListener { void authenticationFailed(String error); void authenticationSuccess(FingerprintManager.AuthenticationResult result); } }
Additionally, we want to create methods to begin authentication, cancel the listner and put into effect the methods in the AuthenticationCallback to interact with our listner.
Integrating Fingerprint authentication, Now our FilgerprintHelper will appear like this.
@RequiresApi(api = Build.VERSION_CODES.M) public class FingerprintHelper extends AuthenticationCallback{ private FingerprintHelperListener listener; public FingerprintHelper(FingerprintHelperListener listener) { this.listener = listener; } private CancellationSignal cancellationSignal; public void startAuth(FingerprintManager manager, FingerprintManager.CryptoObject cryptoObject) { cancellationSignal = new CancellationSignal(); try { manager.authenticate(cryptoObject, cancellationSignal, 0, this, null); } catch (SecurityException ex) { listener.authenticationFailed(“An error occurred: ” + ex.getMessage()); } catch (Exception ex) { listener.authenticationFailed(“An error occurred: ” + ex.getMessage()); } } public void cancel() { if (cancellationSignal != null) cancellationSignal.cancel(); } interface FingerprintHelperListener { void authenticationFailed(String error); void authenticationSuccess(FingerprintManager.AuthenticationResult result); } @Override public void onAuthenticationError(int errorCode, CharSequence errString) { listener.authenticationFailed(“AuthenticationError : “+errString); } @Override public void onAuthenticationHelp(int helpCode, CharSequence helpString) { listener.authenticationFailed(“AuthenticationHelp : “+helpString); } @Override public void onAuthenticationSucceeded(FingerprintManager.AuthenticationResult result) { listener.authenticationSuccess(result); } @Override public void onAuthenticationFailed() { listener.authenticationFailed(“Authentication Failed!”); } }
Implementing the listener in Activity
Once we’ve created the FingerprintHelper.Java and the FingerprintHelperListener interior it, we are able to put into effect the listner in our Activity.
public class LoginActivity extends AppCompatActivity implements FingerprintHelper.FingerprintHelperListener { //Your onCreate and other pieces of code goes here … … //You class should implement the listener and override the below two methods @Override public void authenticationFailed(String error) { //Authentication failed. ie user tried an invalid fingerprint Toast.makeText(this, “Invalid Fingeprint”, Toast.LENGTH_LONG).show(); } @Override public void authenticationSuccess(FingerprintManager.AuthenticationResult result) { //Yaay! we have authenticated the user using Fingerprint. … //add your logic to continue here. } }
We need to create variables of FingerprintManager and FingeprintHelper in our activity.
private FingerprintHelper fingerprintHelper; private FingerprintManager fingerprintManager;
Next, we’ll check for the Fingerprint settings the usage of the function checkFingerprintSettings we discussed above in this post and start listneing while the Activity begins and cancel the listening when the pastime is paused ie. App went to historical past.
Integrating Fingerprint authentication, So upload the subsequent line of code internal your interest via overriding the onResume() and the onPause() method.
@RequiresApi(api = Build.VERSION_CODES.M) @Override protected void onResume() { super.onResume(); //Check for the fingerprint permission and listen for fingerprint //add additional checks along with this condition based on your logic if (checkFingerPrintSettings(this)) { //Fingerprint is available, update the UI to ask user for Fingerprint auth //start listening for Fingerprint fingerprintHelper = new FingerprintHelper(this); fingerprintManager = (FingerprintManager) getSystemService(FINGERPRINT_SERVICE); fingerprintHelper.startAuth(fingerprintManager, null); } else { Log.d(TAG, “Finger print disabled due to No Login credentials or no Fingerprint”); } } @RequiresApi(api = Build.VERSION_CODES.M) @Override protected void onPause() { super.onPause(); if (fingerprintHelper != null) fingerprintHelper.cancel(); }
That’s it! You’re prepared to roll with the new feature on your app. Now your Activity elegance have to appearance some thing like this.
Integrating Fingerprint authentication, Implementing the listener
//loginActivity.java public class LoginActivity extends BaseActivity implements FingerprintHelper.FingerprintHelperListener { //your other variables here // ———————————————– private FingerprintHelper fingerprintHelper; private FingerprintManager fingerprintManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); //Your code for any UI management or custom logic goes here } @RequiresApi(api = Build.VERSION_CODES.M) @Override protected void onResume() { super.onResume(); //Check for the fingerprint permission and listen for fingerprint //add additional checks along with this condition based on your logic if (checkFingerPrintSettings(this)) { //Fingerprint is available, update the UI to ask user for Fingerprint auth //start listening for Fingerprint fingerprintHelper = new FingerprintHelper(this); fingerprintManager = (FingerprintManager) getSystemService(FINGERPRINT_SERVICE); fingerprintHelper.startAuth(fingerprintManager, null); } else { Log.d(TAG, “Finger print disabled due to No Login credentials or no Fingerprint”); } } @RequiresApi(api = Build.VERSION_CODES.M) @Override protected void onPause() { super.onPause(); if (fingerprintHelper != null) fingerprintHelper.cancel(); } @Override public void authenticationFailed(String error) { //Authentication failed. ie user tried an invalid fingerprint Toast.makeText(this, “Invalid Fingeprint”, Toast.LENGTH_LONG).show(); } @Override public void authenticationSuccess(FingerprintManager.AuthenticationResult result) { //Yaay! we have authenticated the user using Fingerprint. … //add your logic to continue here. } /** ** Method to check the availability of Fingerprint on the device ** @param Context context **/ public boolean checkFingerprintSettings(Context context) { final String TAG = “FP-Check”; //Check for android version, FingerPrint is not available below Marshmallow if (Build.VERSION.SDK_INT < Build.VERSION_CODES.M) { Log.d(TAG, “This Android version does not support fingerprint authentication.”); return false; } //Check whether the security option for phone is set. //ie. LockScreen is enabled or not. KeyguardManager keyguardManager = (KeyguardManager) context.getSystemService(KEYGUARD_SERVICE); FingerprintManager fingerprintManager = (FingerprintManager) context.getSystemService(FINGERPRINT_SERVICE); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) { if (!keyguardManager.isKeyguardSecure()) { Log.d(TAG, “User hasn’t enabled Lock Screen”); return false; } } //check if user have registered any fingerprints if (!fingerprintManager.hasEnrolledFingerprints()) { Log.d(TAG, “User hasn’t registered any fingerprints”); return false; } //check for app permissions //Make sure you have mentioned the permission on AndroidManifest.xml if (ActivityCompat.checkSelfPermission(context, Manifest.permission.USE_FINGERPRINT) != PackageManager.PERMISSION_GRANTED) { Log.d(TAG, “User hasn’t granted permission to use Fingerprint”); return false; } Log.d(TAG, “Fingerprint authentication is set.”); return true; } }