Android Fade In / Out Animations, In android, Fade In and Fade Out animations are used to exchange the advent and behaviour of the objects over a specific c programming language of time. The Fade In and Fade Out animations will provide a better appearance and experience for our programs.
Generally, the animations are useful when we need to inform users approximately the alternate’s happening in our app, such as new content material loaded or new moves available, etc.
To create an animation impact to the objects in our android application, we want to observe beneath steps.
Android Fade In / Out Animations,Create XML File to Define Animation
We need to create an xml record that defines the sort of animation to carry out in a brand new folder anim under res listing (res à anim à fade_in.Xml) with required properties. In case anim folder not exists in res listing, create a new one.
To use Fade In or Fade Out animations in our android packages, we need to outline a brand new xml report with tag like as shown below.
For Fade In animation, we need to increase the alpha price from 0 to at least one like as proven under.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<alpha
android:duration="2000"
android:fromAlpha="0.1"
android:toAlpha="1.0">
</alpha>
</set>
For Fade Out animation, we need to decrease the alpha value from 1 to 0 like as shown below.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<alpha
android:duration="2000"
android:fromAlpha="1.0"
android:toAlpha="0.1" >
</alpha>
</set>
Once we are done with creation of required animation XML files, we need to load those animation files using different properties.
Android Fade In / Out Animations,Android Load and Start the Animation
In android, we will carry out animations through using AnimationUtils issue techniques such as loadAnimation(). Following is the code snippet of loading and beginning an animation the use of loadAnimation() and startAnimation() strategies.
ImageView img = (ImageView)findViewById(R.id.imgvw);
Animation aniFade = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.fade_in);
img.startAnimation(aniFade);
If you examine above code snippet, we are including an animation to the photograph the use of loadAnimation() approach. The second parameter in loadAnimation() method is the call of our animation xml record.
Here we used every other technique startAnimation() to use the defined animation to imageview item.
Now we are able to see the way to put in force fade in and fade out animations for imageview on button click on in android packages with examples.
Android Fade In & Fade Out Animations Example
\ Following is the instance of implementing a fade in and fade out animations to vanish in /out photograph on button click in android applications.
Create a brand new android utility using android studio and deliver names as FadeInOutExample. In case in case you aren’t aware about growing an app in android studio check this article Android Hello World App.
Once we create an software, open activity_main.Xml record from reslayout folder course and write the code like as proven under.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="10dp"
android:paddingRight="10dp">
<ImageView android:id="@+id/imgvw"
android:layout_width="wrap_content"
android:layout_height="250dp"
android:src="@drawable/bangkok"/>
<Button
android:id="@+id/btnFadeIn"
android:layout_below="@+id/imgvw"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fade In" android:layout_marginLeft="100dp" />
<Button
android:id="@+id/btnFadeOut"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/btnFadeIn"
android:layout_toRightOf="@+id/btnFadeIn"
android:text="Fade Out" />
</RelativeLayout>
As discussed, we want to create an xml files to define fade in and fade out animations in new folder anim beneath res directory (res à anim à fade_in.Xml, fade_out.Xml) with required houses. In case anim folder no longer exists in res listing, create a brand new one.
Following is the instance of creating an XML files (fade_in.Xml, fade_out.Xml) below anim folder to define fade in / out animation residences.
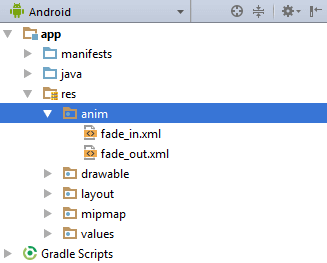
Now open fade_in.xml file and write the code to set fade in animation properties like as shown below.
fade_in.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<alpha
android:duration="2000"
android:fromAlpha="0.1"
android:toAlpha="1.0">
</alpha>
</set>
Now open fade_out.xml file and write the code to set fade out animation properties like as shown below
fade_out.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<alpha
android:duration="2000"
android:fromAlpha="1.0"
android:toAlpha="0.1" >
</alpha>
</set>
Now open your main activity file MainActivity.java from \java\com.tutlane.fadeinoutexample path and write the code like as shown below
MainActivity.java
package com.tutlane.fadeinoutexample;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
private Button btnfIn;
private Button btnfOut;
private ImageView img;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnfIn = (Button)findViewById(R.id.btnFadeIn);
btnfOut = (Button)findViewById(R.id.btnFadeOut);
img = (ImageView)findViewById(R.id.imgvw);
btnfIn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Animation animFadeIn = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.fade_in);
img.startAnimation(animFadeIn);
}
});
btnfOut.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Animation animFadeOut = AnimationUtils.loadAnimation(getApplicationContext(),R.anim.fade_out);
img.startAnimation(animFadeOut);
}
});
}
}
If you look at above code, we’re adding an animation to the picture using loadAnimation() method and used startAnimation() technique to use the defined animation to imageview object.
Output of Android Fade In / Out Animations Example
When we run above program in android studio we will get the result like as shown below.
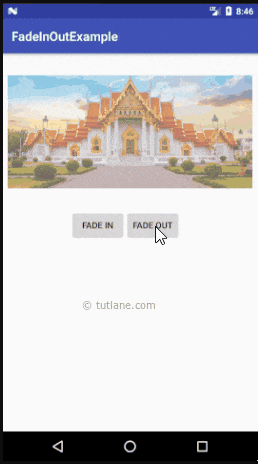
If you study above end result, whenever we are clicking on Fade In or Fade Out buttons, the picture length varies based on our capability.
This is how we will implement fade in and fade in animations for imageview in android packages based on our requirements.