Android Animations,In android, Animations are used to trade the appearance and behavior of the items over a specific c programming language of time. The animations will provide a better appearance and language of time. The animations will provide a better appearance and sense excessive great person interface for our programs.
Generally, the animations are beneficial whilst we need to inform customers about the exchange’s going on in our app, together with new content material loaded or new moves to be had, etc.
To create an animation impact to the gadgets in our android application, we want to observe under steps.
Android Animations,Create XML File to Define Animation
We want to create an xml document that defines the form of animation to carry out in a new folder anim underneath res listing (res à anim à animation.Xml) with required houses.
In case anim folder not exists in res directory, create a new one.
Following is the example of making an XML documents beneath anim folder to outline slide up / down animation properties.
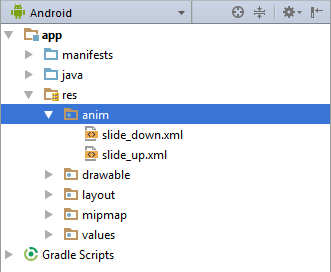
The xml files will contain the code like as shown below based on the type of animation.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="0.0"
android:toXScale="1.0"
android:toYScale="1.0" />
</set>
In case if we want to use distinct form of animations together with fade in / out, zoom in / out, and so forth. We want to create a brand new xml files in anim folder with required houses.
Attributes | Description |
android:duration | It is used to define the duration for the animation to complete. |
android:startOffset | It is used to define the waiting time before an animation starts. |
android:interpolator | It is used to define the rate of change in animation. |
android:repeatMode | It is useful when we want to repeat our animation. |
android:repeatCount | It is used to define the number of times the animation repeats. In case if we set infinite, the animation will repeat infinite times. |
android:fillAfter | It is used to define whether to apply animation transformation after the animation completes or not. |
Android Animations,Android Load and Start the Animation
In android, we are able to perform animations by way of using AnimationUtils element techniques along with loadAnimation(). Following is the code snippet of loading and beginning an animation the use of loadAnimation() and startAnimation() strategies.
ImageView img = (ImageView)findViewById(R.Identification.Imgvw); Animation aniSlide = AnimationUtils.LoadAnimation(getApplicationContext(),R.Anim.Slide_up); img.StartAnimation(aniSlide);
If you have a look at above code snippet, we’re adding an animation to the photograph the usage of loadAnimation() approach. The 2nd parameter in loadAnimation() approach is the name of our animation xml report.
Here we used another approach startAnimation() to apply the described animation to imageview item.
Android Animations,Different Types of Android Animations
Now we are able to see a way to create every animation with required homes in android utility.
Android Animations,Android Fade In / Out Animation
To use Fade In or Fade Out animations in our android applications, we want to outline a brand new xml report with tag like as shown beneath.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<alpha
android:duration="2000"
android:fromAlpha="0.1"
android:toAlpha="1.0">
</alpha>
</set>
For Fade Out animation, we need to decrease the alpha value from 1 to 0 like as shown below.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<alpha
android:duration="2000"
android:fromAlpha="1.0"
android:toAlpha="0.1" >
</alpha>
</set>
Android Slide Up / Down Animation
To use Slide Up or Slide Down animations in our android programs, we need to define a new xml file with tag like as shown below.
For Slide Up animation, we want to set android:fromYScale=”1.0″ and android:toYScale=”0.0″ like as shown beneath.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:toXScale="1.0"
android:toYScale="0.0" />
</set>
For Slide Down animation, we need to set android:fromYScale=”0.0″ and android:toYScale=”1.0″ like as shown below.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/linear_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="0.0"
android:toXScale="1.0"
android:toYScale="1.0" />
</set>
Android Zoom In / Out Animation
To use Zoom In or Zoom Out animations in our android packages, we need to outline a brand new xml report with tag like as proven underneath.
For Zoom In animation, we want to set android:pivotX=”50%” and android:pivotY=”50%” to carry out the zoom from centre of the element.
Also we need to use fromXScale, fromYScale attributes to define the scaling of object and we want preserve those fee lesser than toXScale, toYScale like as proven beneath.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="1000"
android:fromXScale="2"
android:fromYScale="2"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="4"
android:toYScale="4" >
</scale>
</set>
In android, Zoom Out animation is identical as Zoom In animation however fromXScale, fromYScale attribute values ought to be greater than toXScale, toYScale like as proven below.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
android:duration="2500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale=".2"
android:toYScale=".2" />
</set>
To recognise extra about Zoom In and Zoom Out animations check this, Android Zoom In / Out Animations with Examples.
Android Rotate Clockwise / Anti Clockwise Animation
To use Rotate animation in our android programs, we need to outline a brand new xml file with tag like as shown under.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/cycle_interpolator">
<rotate android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:duration="5000" />
</set>
animation in Anti Clockwise, we need to set android:fromDegrees and android:toDegrees property values and these will define a rotation angles like as shown below.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" android:interpolator="@android:anim/cycle_interpolator">
<rotate android:fromDegrees="360"
android:toDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:duration="5000" />
</set>
O recognize greater approximately Rotate animations check this, Android Rotate Clockwise / Anti Clockwise Animations with Examples.
This is how we will use exceptional kinds of animations in android packages based totally on our requirements.