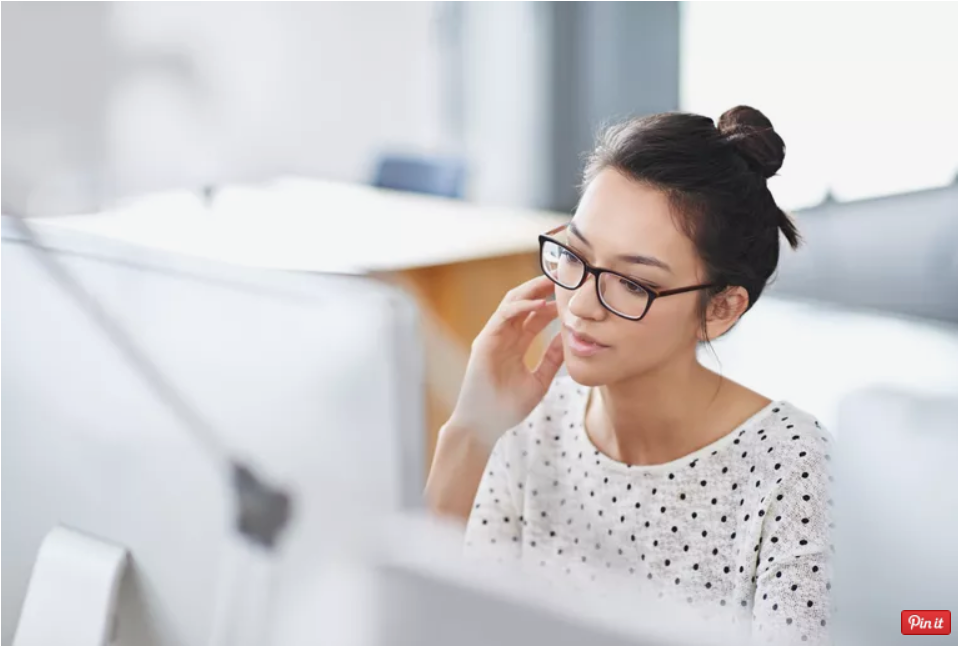
QuickSort Sorting Algorithm, One of the common troubles in programming. Is to kind an array of values in some order (ascending or descending).
To kind an array of values in some order (ascending or descending).
The QuickSort Sorting Algorithm, While there are many “popular” sorting algorithms, QuickSort is one of the quickest.
Quicksort types by using a divide and triumph over strategy to divide a listing into sub-lists.
QuickSort Sorting Algorithm
The primary concept is to pick out. One of the elements in the array known as a pivot. Around the pivot.
Different elements can be rearranged. Everything much less than the pivot is moved left of the pivot.
Into the left partition. Everything extra than the pivot goes into the right partition.
At this point, every partition is recursive “quick taken care of”.
Here’s QuickSort algorithm applied in Delphi:
Proclaims a variable referred to as Appointments that holds a one-dimensional array (vector) of 7 integer values. Given this assertion.
Appointments[3] denotes the fourth integer price in Appointments.
The wide variety within the brackets is known as the index.
If we create a static array but don’t assign values to all its elements, the unused elements incorporate random information.
They may be like uninitialized variables.
The following code may be used to set all elements within the Appointments array to zero.
procedure QuickSort(var A: array of Integer; iLo, iHi: Integer) ;
var
Lo, Hi, Pivot, T: Integer;
begin
Lo := iLo;
Hi := iHi;
Pivot := A[(Lo + Hi) div 2];
repeat
while A[Lo] < Pivot do Inc(Lo) ;
while A[Hi] > Pivot do Dec(Hi) ;
if Lo <= Hi then
begin
T := A[Lo];
A[Lo] := A[Hi];
A[Hi] := T;
Inc(Lo) ;
Dec(Hi) ;
end;
until Lo > Hi;
if Hi > iLo then QuickSort(A, iLo, Hi) ;
if Lo < iHi then QuickSort(A, Lo, iHi) ;
end;
Usage :
var
intArray : array of integer;
begin
SetLength(intArray,10) ;
//Add values to intArray
intArray[0] := 2007;
...
intArray[9] := 1973;
//sort
QuickSort(intArray, Low(intArray), High(intArray)) ;
Note: in practice, the QuickSort turns into very slow whilst the array exceeded to it’s miles already close to being taken care of.
There’s a demo software that ships with Delphi.
Referred to as “thrddemo” in the “Threads” folder.
Which shows extra sorting algorithms: Bubble sort and Selection Sort.
Very regularly, your software doesn’t understand at compile time.
What number of elements could be wanted.
That range will now not be acknowledged till runtime.
With dynamic arrays, you could allocate handiest as a lot storage.
As is required at a given time. In other phrases.
The scale of dynamic arrays can be modified at runtime.
Which is one of the key blessings of dynamic arrays.
The subsequent instance creates an array of integer values and then calls.
The Copy characteristic to resize the array.