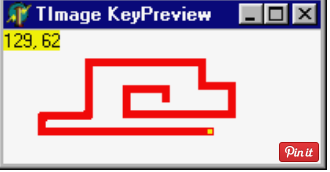
Keyboard,Zarko Gajic is experienced in SQL and has operating expertise of DB structures consisting of MS SQL Server, Firebird, Interbase, and Oracle. He is likewise proficient in XML, DHTML, and JavaScript.
Keyboard Input Intercept
Most Delphi packages normally take care of user enter thru specific occasion handlers, people who enable us to seize user keystrokes and process mouse movement.
We understand that awareness is the capacity to receive consumer input via the mouse or keyboard. Only the object that has the point of interest can obtain a keyboard event. Some controls, such as TImage, TPaintBox, TPanel, and TLabel cannot acquire recognition. The number one purpose of maximum photo controls is to show text or photographs.
If we want to intercept keyboard input for controls that can not receive the enter recognition we’re going to ought to address Windows API, hooks, callbacks and messages.
Keyboard Windows Hooks
Technically, a “hook” characteristic is a callback feature that can be inserted in the Windows message machine so an utility can get right of entry to the message circulate earlier than other processing of the message takes vicinity.
Among many sorts of home windows hooks, a keyboard hook is called on every occasion the application calls the GetMessage() or PeekMessage() characteristic and there is a WM_KEYUP or WM_KEYDOWN keyboard message to method.
To create a keyboard hook that intercepts all keyboard input directed to a given thread, we need to name SetWindowsHookEx API function. The exercises that obtain the keyboard activities are application-defined callback functions referred to as hook features (KeyboardHookProc).
Windows calls your hook characteristic for every keystroke message (key up and key down) before the message is placed within the application’s message queue. The hook function can system, alternate or discard keystrokes. Hooks may be nearby or international.
The return fee of SetWindowsHookEx is a cope with to the hook just mounted. Before terminating, an software should name the UnhookWindowsHookEx function to loose system resources associated with the hook.
Keyboard Hook Example
As an illustration of keyboard hooks, we’re going to create a project with graphical control that can receive key presses.TImage is derived from TGraphicControl, it could be used as a drawing floor for our hypothetical warfare game.
Since TImage is unable to get hold of presses via trendy events we’re going to create a hook function that intercepts all input directed to our drawing surface.
TImage Processing Events
Start new Delphi Project and place one Image thing on a shape. Set Image1.Align assets to alClient. That’s it for the visible part, nowKeyboard we ought to do a little coding. First, we will need a few global variables:
var
Form1: TForm1;
KBHook: HHook; {this intercepts keyboard input}
cx, cy : integer; {track battle ship's position}
{callback's declaration}
function KeyboardHookProc(Code: Integer; WordParam: Word; LongParam: LongInt): LongInt; stdcall;
implementation
...
To installation a hook, we name SetWindowsHookEx inside the OnCreate occasion of a shape.
procedure TForm1.FormCreate(Sender: TObject) ;
begin
{Set the keyboard hook so we can intercept keyboard input}
KBHook:=SetWindowsHookEx(WH_KEYBOARD,
{callback >} @KeyboardHookProc,
HInstance,
GetCurrentThreadId()) ;
{place the battle ship in the middle of the screen}
cx := Image1.ClientWidth div 2;
cy := Image1.ClientHeight div 2;
Image1.Canvas.PenPos := Point(cx,cy) ;
end;
To free gadget sources associated with the hook, we should name the UnhookWindowsHookEx function within the OnDestroy occasion:
procedure TForm1.FormDestroy(Sender: TObject) ;
begin
{unhook the keyboard interception}
UnHookWindowsHookEx(KBHook) ;
end;
The most important part of this project is the KeyboardHookProc callback procedureused to process keystrokes.
function KeyboardHookProc(Code: Integer; WordParam: Word; LongParam: LongInt) : LongInt;
begin
case WordParam of
vk_Space: {erase battle ship's path}
begin
with Form1.Image1.Canvas do
begin
Brush.Color := clWhite;
Brush.Style := bsSolid;
Fillrect(Form1.Image1.ClientRect) ;
end;
end;
vk_Right: cx := cx+1;
vk_Left: cx := cx-1;
vk_Up: cy := cy-1;
vk_Down: cy := cy+1;
end; {case}
If cx < 2 then cx := Form1.Image1.ClientWidth-2;
If cx > Form1.Image1.ClientWidth -2 then cx := 2;
If cy < 2 then cy := Form1.Image1.ClientHeight -2 ;
If cy > Form1.Image1.ClientHeight-2 then cy := 2;
with Form1.Image1.Canvas do
begin
Pen.Color := clRed;
Brush.Color := clYellow;
TextOut(0,0,Format('%d, %d',[cx,cy])) ;
Rectangle(cx-2, cy-2, cx+2,cy+2) ;
end;
Result:=0;
{To prevent Windows from passing the keystrokes to the target window, the Result value must be a nonzero value.}
end;
That’s it. We now have the ultimate keyboard processing code.
Note just one factor: this code is in no manner constrained for use only with TImage.
The KeyboardHookProc characteristic serves as a popular KeyPreview & KeyProcess mechanism.