All programming languages support comments which are ignored by the compiler
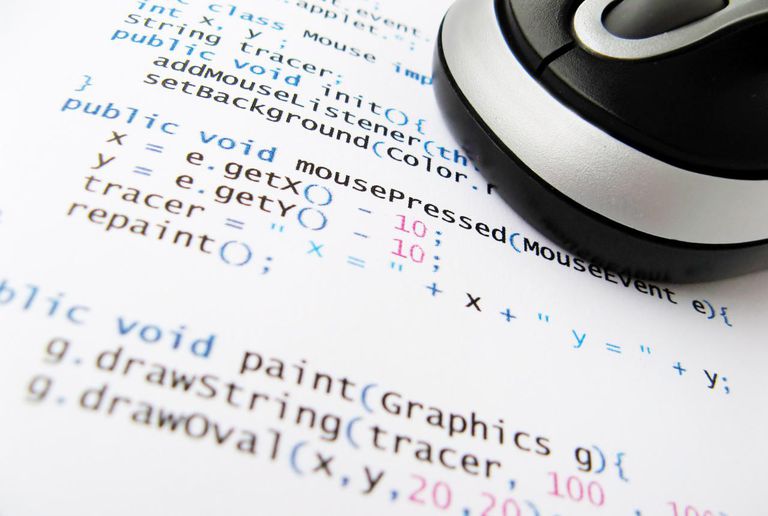
Java Comments, feedback are notes in a Java code file which are unnoticed with the aid of the compiler and runtime engine. They are used to annotate the code so that it will clarify its design and motive.
You can add a vast quantity of remarks to a Java file, however there are some “best practices” to observe whilst using feedback.
Generally, code comments are “implementation” feedback that specify the source code, inclusive of descriptions of classes, interfaces, techniques, and fields. These are commonly a couple of lines written above or beside Java code to make clear what it does.
Another form of Java comment is a Javadoc comment. Javadoc remarks range barely in syntax from implementation comments and are used by this system javadoc.Exe to generate Java HTML documentation.
Why Use Java Comments?
It’s top practice to get into the addiction of placing Java remarks into your supply code to decorate its clarity and readability for yourself and other programmers. It isn’t always instantly clear what a phase of Java code is performing. A few explanatory traces can drastically lessen the amount of time it takes to apprehend the code.
Do They Affect How the Program Runs?
Implementation feedback in Java code are best there for people to examine. Java compilers do not care about them and while compiling this system, they simply pass over them. The size and efficiency of your compiled software will no longer be tormented by the variety of feedback for your source code.
Java Comments, Implementation Comments
Implementation comments come in two exceptional formats:
- Line Comments: For a one line comment, type “//” and observe the 2 ahead slashes together with your comment. For instance:
// this is a single line comment int guessNumber = (int) (Math.random() * 10);
When the compiler comes throughout the two forward slashes, it knows that the whole thing to the right of them is to be considered as a comment. This is beneficial when debugging a bit of code. Just upload a comment from a line of code you are debugging, and the compiler won’t see it:
// this is a single line comment // int guessNumber = (int) (Math.random() * 10);
You can also use the two forward slashes to make an end of line comment:
// this is a single line comment int guessNumber = (int) (Math.random() * 10); // An end of line comment
- Block Comments: To begin a block remark, type “/“. Everything among the forward shrink and asterisk, despite the fact that it’s on a one of a kind line, is treated as a remark until the characters “/” give up the comment. For instance:
/* this
is
a
block
comment
*/
/* so is this */
Javadoc Comments
Use special Javadoc remarks to file your Java API. Javadoc is a tool protected with the JDK that generates HTML documentation from feedback in source code.
A Javadoc remark in
.java
source files is enclosed in start and end syntax like so:
/**
and
*/
. Each comment within these is prefaced with a
*
Place those comments at once above the technique, elegance, constructor or some other Java element which you want to report. For example:
// myClass.java
/**
* Make this a summary sentence describing your class.
* Here's another line.
*/
public class myClass
{
...
}
Javadoc incorporates various tags that control how the documentation is generated. For example, the
@param
tag defines parameters to a method:
/** main method
* @param args String[]
*/
public static void main(String[] args)
{
System.out.println("Hello World!");
}
Many other tags are available in Javadoc, and it also supports HTML tags to help manipulate the output. See your Java documentation for greater detail.
Tips for Using Comments
- Don’t over remark. Every line of your application does not want to be explained. If your application flows logically and nothing sudden takes place, do not feel the need to add a comment.
- Indent your comments. If the line of code you are commenting is indented, make sure your comment suits the indentation.
- Keep comments applicable. Some programmers are amazing at editing code, but for some cause forget to update the feedback. If a remark now not applies, then either modify or put off it.
- Don’t nest block feedback. The following will bring about a compiler error:
/* this
is
/* This block comment finishes the first comment */
a
block
comment
*/