Firebase Authentication with Google, is a cell and net application platform with tools and infrastructure designed to assist developers construct great apps.
Firebase is made from complementary capabilities that developers can blend-and-healthy to in shape their needs.
Services provided through Firebase which could help developers build and release programs faster encompass:
- Cloud Messaging
- Authentication
- Realtime Database
- Storage
- Hosting
- Crash Reporting to keep your apps stable and free from bugs.
- Test Lab to deliver high quality apps.
This academic goes to recognition on the Authentication carrier furnished by way of Firebase the use of Google SignIn and Firebase Realtime Database in Android Applications.
Authentication is the system of identifying an individual, typically based totally on a username and password earlier than they are able to access certain assets in our case cellular software sources like information and other statistics (Authentication is truly the SignIn Process for Apps)
Firebase Realtime database is a NoSQL Cloud database that synced records across all customers for example, net, mobile and different customers and this happens in actual time and even while the customer is offline, the facts stays to be had.
How to add Firebase Google Login For Authentication.
Follow the below steps to do this:
01. Visit the Firebase Website firebase.Google.Com and create a firebase account first of all. Go to Firebase console and click the “Create New Project” Button.
02. The Create a challenge window seems and you’re required to go into your challenge call and your Country/Region, Click Create assignment to proceed.
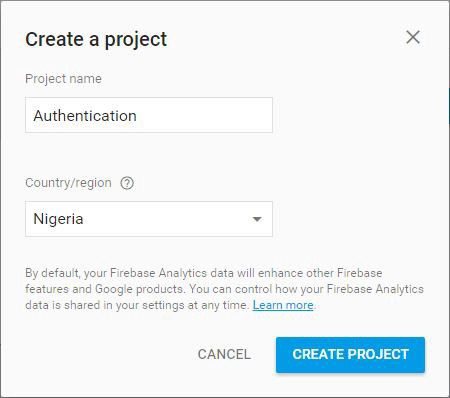
03. In the subsequent display choose “Add Firebase on your Android App” after which add the bundle info and Debug signing certificates SHA-1 key. Click right here for a tutorial on Getting Android Debug Keystore SHA1 Fingerprint:
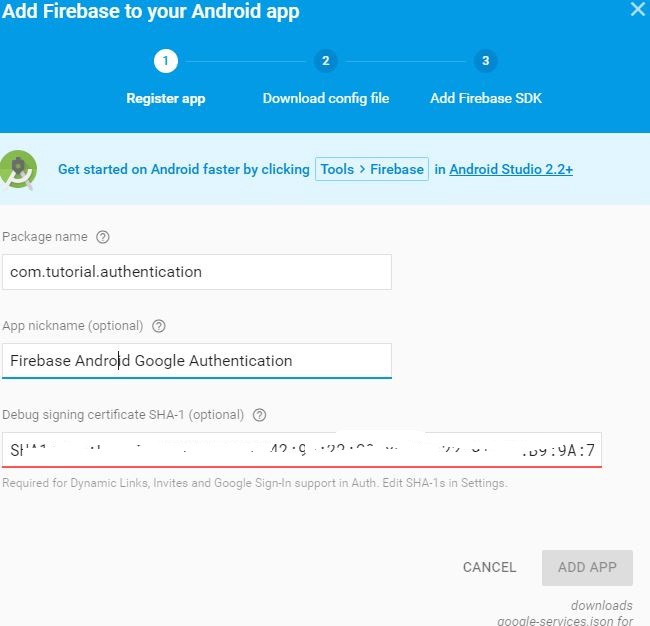
After clicking ADD APP, in the next web page, you will be requested to download the google-services.Json record. Please, download it in your pc. We will upload it to our android app later. After this, you’ll be taken in your challenge Dashboard.
04. In the project Dashboard, click the Authentication in the Menu at the left of the page, then click on the SIGN-IN METHOD, you’ll be taken to a web page in which you may allow and disable unique SignIn providers that Firebase Support, go ahead and Enable Google SignIn and then click store.
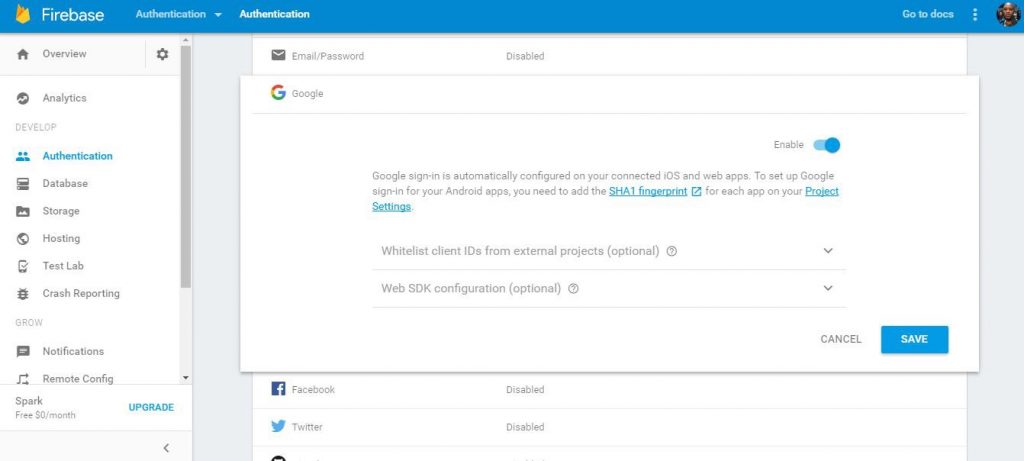
05. Create Android Firebase Login App in Android Studio.
Let’s create the Android Application a good way to hook up with Firebase for consumer authentication using Firebase Google Login.
Steps to creating a project on Android Studio
- Launch Android Studio and Go to File → New → New Project and enter your Application Name.
- Enter agency domain, that is used to uniquely pick out your App’s package deal international. Remember to use the equal package name as used inside the firebase console.
- Choose assignment region and minimal SDK and on the subsequent display choose Empty Activity, Then Click on Next.
- Choose an Activity Name. Make certain Generate Layout File check container is chosen, Otherwise we must generate it ourselves.Then click on on Finish. We have used the Activity Name as MainActivity considering the fact that this could be the default display when the consumer opens the app for the primary time.
Gradle will configure and construct your challenge and remedy the dependencies, Once it is entire proceed for subsequent steps.
Add Permissions and Dependencies
01. After Gradle syncs the task, upload the google-offerings.Json file that you downloaded at the same time as growing a brand new project at the Firebase console in your venture’s app folder as shown beneath.
You can drag the file and positioned it in the Android app module folder without delay into android studio. Or you can surely replica the document and paste it inside the Android Studio Project App directory to your laptop.
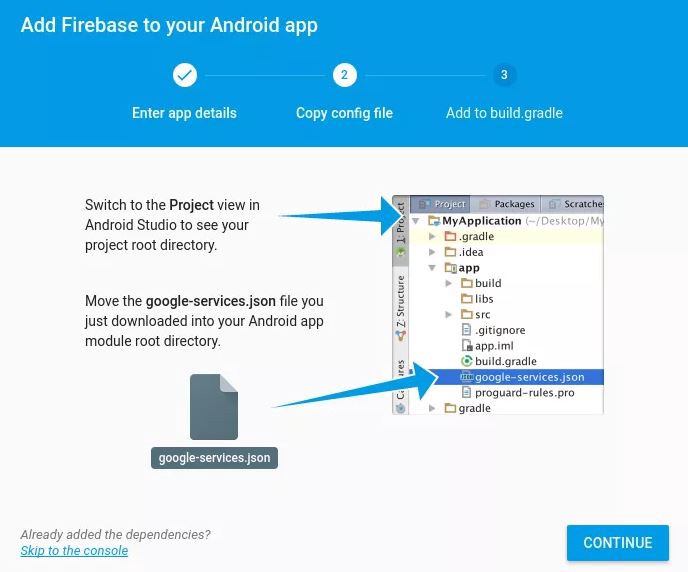
02. Since we want to hook up with the Network at the same time as authenticating, you’ll need to feature the Internet permission for your AndroidManifest.Xml document that’s more like a configuration document to your App.
AndroidManifest.xml file
<uses-permission android:name=”android.permission.INTERNET”/>
Open your project’s build.Gradle file from the venture’s domestic directory and add the following dependency. Please note that i’m no longer speaking approximately the module or app’s construct.Gradle file, we’ll get to that in the subsequent step
Build.gradle file
classpath ‘com.google.gms:google-services:3.0.0’
04. Next, open your app’s build.Gradle from the and add the following on the quit of the record.
Build.Gradle
apply plugin: ‘com.google.gms.google-services’
05. Also upload the subsequent dependency inside the dependency segment.
compile ‘com.google.firebase:firebase-core:10.2.0’
compile ‘com.google.firebase:firebase-auth:10.2.0’
compile ‘com.google.android.gms:play-services-auth:10.2.0’
After including those dependencies, Android Studio could want to sync, ensure you are connected to the net so Android Studio can download those used dependencies.
So allow’s start coding the functionalities:
Open activity_main.Xml document in the res/format folder and add the subsequent code. Since we are going to be showing the username and e-mail of the signed in user in a NavigationDrawer header view, we’ll just have most effective the Custom Google SignIn Button in this layout.
<?xml version=”1.0" encoding=”utf-8"?>
<LinearLayout xmlns:android=”http://schemas.android.com/apk/res/android"
xmlns:tools=”http://schemas.android.com/tools"
android:layout_width=”match_parent”
android:layout_height=”match_parent”
android:paddingBottom=”@dimen/activity_vertical_margin”
android:paddingLeft=”@dimen/activity_horizontal_margin”
android:paddingRight=”@dimen/activity_horizontal_margin”
android:paddingTop=”@dimen/activity_vertical_margin”
android:orientation=”vertical”
android:gravity=”center”
tools:context=”com.tutorial.authentication.MainActivity”>
<com.google.android.gms.common.SignInButton
android:id=”@+id/login_with_google”
android:layout_width=”match_parent”
android:layout_height=”wrap_content” />
</LinearLayout>
Now open your MainActivity.Java in the App/Java folder, create a technique called configureSignIn, outdoor the onCreate() method and positioned within the below codes.
Add Permissions and Dependencies
Then name that method inside the onCreate approach of the class. Please, click on here to view the venture on github, check there for different resources required to build this utility.
For instance the MainActivity.Magnificence extends every other elegance referred to as BaseActivity as a way to be written short.
We want to configure Google Sign-In so one can be able to get the person information. So, we create a GoogleSignInOptions item with the requestEmail option.
Add this code to the MainActivity Class:
// This method configures Google SignIn
public void configureSignIn(){
// Configure sign-in to request the user’s basic profile like name and email
GoogleSignInOptions options = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestEmail()
.build();
// Build a GoogleApiClient with access to GoogleSignIn.API and the options above.
mGoogleApiClient = new GoogleApiClient.Builder(this)
.enableAutoManage(this /* FragmentActivity */, this /* OnConnectionFailedListener */)
.addApi(Auth.GOOGLE_SIGN_IN_API, options)
.build();
}
Next, Add a signIn() method so one can be known as when the Sign In Button is clicked in the view, this may spark off the consumer to choose a Google account before the authentication system starts offevolved. This technique will handle signal-in by growing a sign-in purpose with the getSignInIntent() approach, and starting the motive with startActivityForResult().
private void signIn() {
Intent signInIntent = Auth.GoogleSignInApi.getSignInIntent(mGoogleApiClient);
startActivityForResult(signInIntent, RC_SIGN_IN);
}
Please be aware that if you don’t have other prolonged or all the assets for this mission, you’ll be getting a few errors by means of just running the piece of codes in this text.
So it’s right to both open the challenge supply code down even as analyzing this educational or after studying, make certain you test out the venture REPO ON GITHUB.
Some instance variables are declared inside the class as an instance mGoogleApiClient and others.
After the person selects an account and the technique of authentication with Google begins, the end result could be made to be had within the onActivityResult() as stated within the piece of code beneath.
When the signIn button is clicked, the person will first be authenticated with Google and if this is successful, we’ll now authenticate the person with Firebase after saving person facts to SharedPreference.
Add Permissions and Dependencies
So we are able to have access to them for show within the Header view of our Navigation Drawer Activity. So it’s a 2 stage authentication, the first stage is with Google and the Second with Firebase. I guess this presents a double and comfy authentication system.
// This IS the method where the result of clicking the signIn button will be handled
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// Result returned from launching the Intent from GoogleSignInApi.getSignInIntent(…);
if (requestCode == RC_SIGN_IN) {
GoogleSignInResult result = Auth.GoogleSignInApi.getSignInResultFromIntent(data);
if (result.isSuccess()) {
// Google Sign In was successful, save Token and a state then authenticate with Firebase
GoogleSignInAccount account = result.getSignInAccount();
idToken = account.getIdToken();
name = account.getDisplayName();
email = account.getEmail();
photoUri = account.getPhotoUrl();
photo = photoUri.toString();
// Save Data to SharedPreference
sharedPrefManager = new SharedPrefManager(mContext);
sharedPrefManager.saveIsLoggedIn(mContext, true);
sharedPrefManager.saveEmail(mContext, email);
sharedPrefManager.saveName(mContext, name);
sharedPrefManager.savePhoto(mContext, photo);
sharedPrefManager.saveToken(mContext, idToken);
//sharedPrefManager.saveIsLoggedIn(mContext, true);
AuthCredential credential = GoogleAuthProvider.getCredential(idToken, null);
firebaseAuthWithGoogle(credential);
} else {
// Google Sign In failed, update UI appropriately
Log.e(TAG, “Login Unsuccessful. “);
Toast.makeText(this, “Login Unsuccessful”, Toast.LENGTH_SHORT)
.show();
}
}
}
Next, after authenticating with Google, it’s now time to authenticate with Firebase. Before we continue, we need to claim the example of FirebaseAuth item and AuthStateListener which listens for adjustments within the signed in user state.
So claim those as instance variables:
private FirebaseAuth mAuth;
private FirebaseAuth.AuthStateListener mAuthListener;
Then, in the onCreate() technique, we’ll want to initialize the FirebaseAuth instance and the AuthStateListener approach so we can concentrate and music whether or not the user is signed in or out.
mAuth = com.google.firebase.auth.FirebaseAuth.getInstance();
//this is where we start the Auth state Listener to listen for whether the user is signed in or not
mAuthListener = new FirebaseAuth.AuthStateListener(){
@Override
public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) {
// Get signedIn user
FirebaseUser user = firebaseAuth.getCurrentUser();
//if user is signed in, we call a helper method to save the user details to Firebase
if (user != null) {
// User is signed in
createUserInFirebaseHelper();
Log.d(TAG, “onAuthStateChanged:signed_in:” + user.getUid());
} else {
// User is signed out
Log.d(TAG, “onAuthStateChanged:signed_out”);
}
}
};
If the user is effectively authenticated by means of Firebase, we’ll now call the createUserInFirebaseHelper(); approach that saves the user data to our own Firebase Database.
This approach of saving the consumer records to our very own Firebase is a good practice after authentication so we are able to have our own copy of the users details persevered to Firebase database. So from onAuthStateChanged technique, we’ll name a helper technique so one can now take the user details in particular username, photograph URL and email after which save them to Firebase Database.
Firebase Authentication with Google, Add Permissions and Dependencies
So before you observe the createUserInFirebaseHelper() technique that saves information to Firebase, i want you to understand that we might be going outdoor the scope of this academic that is truly Authentication with Google and Firebase. Now, we are dabbing into placing information into Firebase database, which is a topic of it’s own. I’m simply enforcing this here as an awesome exercise but there is more you need to know about Inserting and selecting from Firebase database. I desire to in my subsequent article, write a step by step manual on inserting and selecting from Firebase.
You want to have the URL on your Firebase database, add a listener to the location and onDataChange. You insert the facts by using passing an object containing the statistics you want to store. Please don’t get pressured here. I promise to do an article as said above with a view to deal drastically on this.
//This method creates a new user on our own Firebase database
//after a successful Authentication on Firebase
//It also saves the user info to SharedPreference
private void createUserInFirebaseHelper(){
//Since Firebase does not allow “.” in the key name, we’ll have to encode and change the “.” to “,”
// using the encodeEmail method in class Utils
final String encodedEmail = Utils.encodeEmail(email.toLowerCase());
//create an object of Firebase database and pass the the Firebase URL
final Firebase userLocation = new Firebase(Constants.FIREBASE_URL_USERS).child(encodedEmail);
//Add a Listerner to that above location
userLocation.addListenerForSingleValueEvent(new com.firebase.client.ValueEventListener() {
@Override
public void onDataChange(com.firebase.client.DataSnapshot dataSnapshot) {
if (dataSnapshot.getValue() == null){
/* Set raw version of date to the ServerValue.TIMESTAMP value and save into dateCreatedMap */
HashMap<String, Object> timestampJoined = new HashMap<>();
timestampJoined.put(Constants.FIREBASE_PROPERTY_TIMESTAMP, ServerValue.TIMESTAMP);
// Insert into Firebase database
User newUser = new User(name, photo, encodedEmail, timestampJoined);
userLocation.setValue(newUser);
Toast.makeText(MainActivity.this, “Account created!”, Toast.LENGTH_SHORT).show();
// After saving data to Firebase, goto next activity
Intent intent = new Intent(MainActivity.this, NavDrawerActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
finish();
}
}
@Override
public void onCancelled(FirebaseError firebaseError) {
Log.d(TAG, getString(R.string.log_error_occurred) + firebaseError.getMessage());
//hideProgressDialog();
if (firebaseError.getCode() == FirebaseError.EMAIL_TAKEN){
}
else {
Toast.makeText(MainActivity.this, firebaseError.getMessage(), Toast.LENGTH_SHORT).show();
}
}
});
}
Next, after correctly inserting into our Firebase database, we move to the second Activity wherein we’ll display consumer data. The under piece of code from the above approach actions us to the second one Activity:
/ After saving data to Firebase, goto next activity
Intent intent = new Intent(MainActivity.this, NavDrawerActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
finish();
Don’t neglect the component that kicks of this entire system that’s setting an onClicklisterner at the Google signIn Button. Whilst this button is clicked, the signIn() approach is referred to as and the consumer is prompted to select an account:
mSignInButton = (SignInButton) findViewById(R.id.login_with_google);
mSignInButton.setSize(SignInButton.SIZE_WIDE);
mSignInButton.setOnClickListener(this);
@Override
public void onClick(View view) {
Utils utils = new Utils(this);
int id = view.getId();
if (id == R.id.login_with_google){
if (utils.isNetworkAvailable()){
signIn();
}else {
Toast.makeText(MainActivity.this, “Oops! no internet connection!”, Toast.LENGTH_SHORT).show();
}
}
}
Next, in the onCreate() method of the second one Activity, we get the records saved from SharedPreference. SharedPreference stores information as key cost pairs inside the utility Context and makes it available within that software however loses its information while the user logs out of the app, that’s why we are saving our very own copy in our database.
Firebase Authentication with Google, Add Permissions and Dependencies
The underneath code receives a take care of to the perspectives inside the layout. Let’s data from sharedPreferences and sets them to the Header view of the Navigation for show. Please check the GITHUB REPO for the whole lessons and other sources.
mFullNameTextView = (TextView) header.findViewById(R.id.fullName);
mEmailTextView = (TextView) header.findViewById(R.id.email);
mProfileImageView = (CircleImageView) header.findViewById(R.id.profileImage);
// create an object of sharedPreferenceManager and get stored user data
sharedPrefManager = new SharedPrefManager(mContext);
mUsername = sharedPrefManager.getName();
mEmail = sharedPrefManager.getUserEmail();
String uri = sharedPrefManager.getPhoto();
Uri mPhotoUri = Uri.parse(uri);
mFullNameTextView.setText(mUsername);
mEmailTextView.setText(mEmail);
Picasso.with(mContext)
.load(mPhotoUri)
.placeholder(android.R.drawable.sym_def_app_icon)
.error(android.R.drawable.sym_def_app_icon)
.into(mProfileImageView);
Next, the below code in the second activity, set’s up the NavigationDrawer and the data is displayed on the Header View of the NavigationDrawer:
// Initialize and add Listener to NavigationDrawer
public void initNavigationDrawer(){
navigationView = (NavigationView) findViewById(R.id.navigation_view);
navigationView.setNavigationItemSelectedListener(new NavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id){
case R.id.freebie:
Toast.makeText(getApplicationContext(),”Home”,Toast.LENGTH_SHORT).show();
drawerLayout.closeDrawers();
break;
case R.id.payment:
Toast.makeText(getApplicationContext(),”Settings”,Toast.LENGTH_SHORT).show();
drawerLayout.closeDrawers();
break;
case R.id.trip:
Toast.makeText(getApplicationContext(),”Trash”,Toast.LENGTH_SHORT).show();
drawerLayout.closeDrawers();
break;
case R.id.logout:
signOut();
drawerLayout.closeDrawers();
break;
case R.id.tips:
Toast.makeText(getApplicationContext(),”Trash”,Toast.LENGTH_SHORT).show();
drawerLayout.closeDrawers();
break;
}
return false;
}
});
//set up navigation drawer
drawerLayout = (DrawerLayout) findViewById(R.id.drawer);
ActionBarDrawerToggle actionBarDrawerToggle = new ActionBarDrawerToggle(this,drawerLayout, toolbar, R.string.drawer_open, R.string.drawer_close){
@Override
public void onDrawerClosed(View drawerView) {
super.onDrawerClosed(drawerView);
}
@Override
public void onDrawerOpened(View drawerView) {
super.onDrawerOpened(drawerView);
}
};
drawerLayout.addDrawerListener(actionBarDrawerToggle);
actionBarDrawerToggle.syncState();
}
Finally, we create the SignOut method to enable user logout of the app:
//method to logout
private void signOut(){
new SharedPrefManager(mContext).clear();
mAuth.signOut();
Auth.GoogleSignInApi.revokeAccess(mGoogleApiClient).setResultCallback(
new ResultCallback<Status>() {
@Override
public void onResult(@NonNull Status status) {
Intent intent = new Intent(NavDrawerActivity.this, MainActivity.class);
startActivity(intent);
}
}
);
}
Now, run the app and click on on Google Sign In Button to authenticate the usage of Firebase Google Login. It must show a popup with available google account Click on your google account.
And the Firebase Google Login App have to display your Display name. Image and Email Address on the Header View of the Navigation Drawer inside the 2nd pastime.