Android Push Notifications,In android, Push Notification is used to send an essential messages to the cellular gadgets about our cellular app. By the usage of Push Notifications we will allow human beings know approximately the events happening in our app by way of sending a notifications to the cell devices which incorporates our app. For instance, purchasing app’s with a purpose to send a notifications approximately the sale happening of their web sites.
In android, via the usage of Firebase Cloud Messaging (FCM) we will effortlessly push a notifications to the mobile devices with a view to comprise our cell app.
Android Push Notifications,What is Firebase Cloud Messaging?
Firebase Cloud Messaging (FCM) is a go platform (Android, iOS, Mobile Web) messaging answer which is used to send notification messages to the cell devices for free of charge.
By the use of firebase we are able to effortlessly send messages to any device or schedule a messages to ship in consumer’s nearby time region based on our requirements.
We don’t need to write down much coding to ship a notifications and firebase will offer an in depth engagement and conversion tracking for notification messages.
Following is the pictorial representation of how firebase will work with android applications to ship or receive messages.
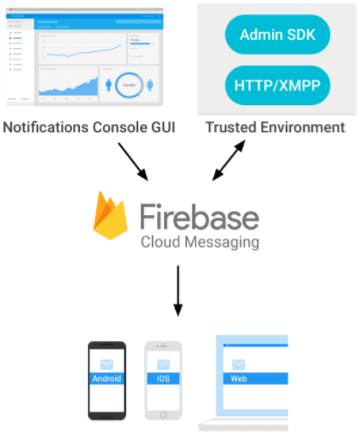
Now we will see how to use firebase cloud messaging in our android programs to push notifications based on our necessities.
Android Push Notifications, Example
Create a new android software using android studio and give names as PushNotification. In case if you are not aware of growing an app in android studio check this article Android Hello World App.
Once we are done with advent of latest app, we want to combine a Firebase in our android packages to push notifications.
Android Push Notifications,Add Firebase to our Android App
To upload Firebase to our android app, first we want to create a Firebase project and Firebase configuration report for our app.
To create a Firebase challenge. Open Firebase Console and it will ask you to login with Google mail account.when we’re logged in, select Add Project like as shown under.
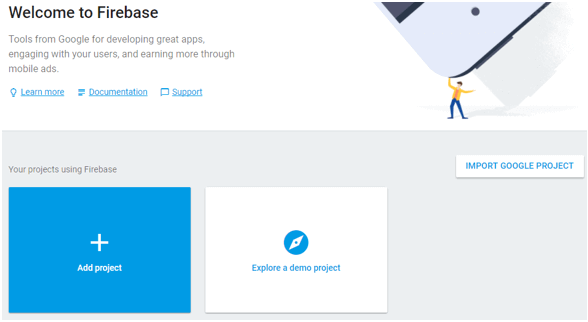
when we click on on Add Project, a brand new popup will open in that input required information like assignment call, usa and click on on Create Project like as proven beneath.
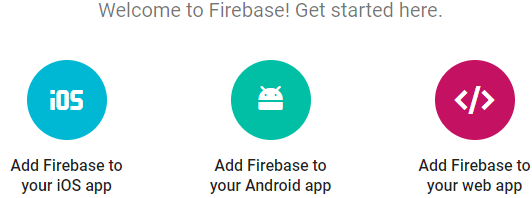
Once we pick out the desired platform, a new popup will open in that we need to go into our app package deal name. This package deal name should be equal as which we described in our android AndroidMaifest.Xml record and click Register App button like as proven below.
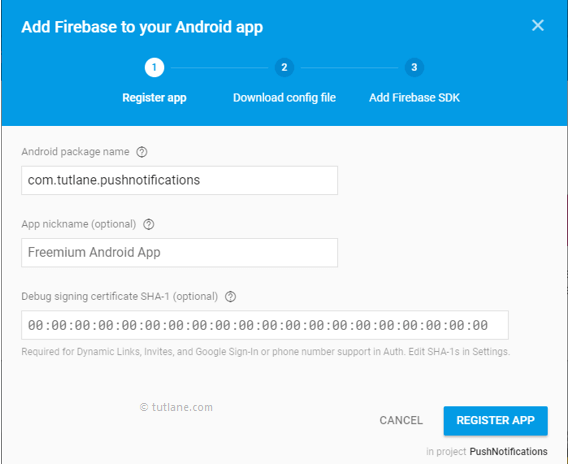
Once we check in our app, we can get google-services.Json record to down load and add it to our assignment app folder like as shown below.
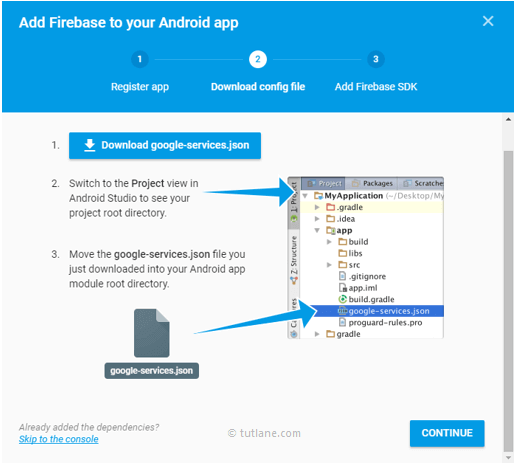
Once we add the downloaded google-offerings.Json record in our assignment app folder so one can be like as proven below.
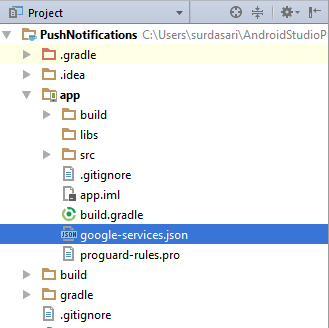
Now we want to adjust our software construct.Gradle document in undertaking degree (/build.Gradle) to include our firebase plugin report (google-services.Json) like as shown below.
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
jcenter()
}
dependencies {
classpath 'com.android.tools.build:gradle:2.3.3'
// Add this line
classpath 'com.google.gms:google-services:3.1.0'
}
}
Now open app level build.gradle (<project>/<app-module>/build.gradle) and make following changes at the bottom of file.
apply plugin: 'com.android.application'
android {
….
}
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
….
// Add this line
compile 'com.google.firebase:firebase-messaging:9.4.0' }
// Add this line to the bottom of file
apply plugin: 'com.google.gms.google-services'
After including the desired dependencies click on Sync Now alternative in the proper facet to sync our venture with required files.
Once we’re carried out with registering our app with firebase, we want to combine firebase cloud messaging in our android application to ship notifications.
Android Push Notifications,Integrate Firebase Cloud Messaging
To integrate FCM (firebase cloud messaging) in android app, we want to create a new magnificence file FireBaseMessaging.Java in javacom.Tutlane.Pushnotifications course and write the code like as proven beneath.
FireBaseMessaging.java
package com.tutlane.pushnotifications;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Context;
import android.content.Intent;
import android.media.RingtoneManager;
import android.net.Uri;
import android.support.v4.app.NotificationCompat;
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
/**
* Created by surdasari on 21-09-2017.
*/
public class FireBaseMessaging extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage rMsg) {
sendNotification(rMsg.getNotification());
}
private void sendNotification(RemoteMessage.Notification rNotfy) {
Uri soundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
Intent rintent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, rintent, 0);
NotificationCompat.Builder builder = new NotificationCompat.Builder(this)
.setContentTitle(rNotfy.getTitle())
.setContentText(rNotfy.getBody())
.setAutoCancel(true)
.setSmallIcon(R.drawable.ic_notification)
.setSound(soundUri)
.setContentIntent(pendingIntent);
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.notify(0, builder.build());
}
}
If you have a look at above code, we’re extending our class (FireBaseMessaging.Java) file behaviour the use of FirebaseMessagingService magnificence. The FirebaseMessagingService elegance is the bottom class to talk with firebase messaging and it provide a capability to show the notifications routinely.
Now we need to sign in our newly created elegance files in AndroidManifest.Xml record like as shown under
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.tutlane.pushnotifications">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service
android:name=".FireBaseMessaging">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
</application>
</manifest>
If you take a look at above code, we registered our FireBaseMessaging class in AndroidManifest.Xml record and introduced a permission to access INTERNET because firebase cloud messaging required an INTERNET access to expose the notifications.
Generally, for the duration of the release of our activity, onCreate() callback method can be known as by using android framework to get the required layout for an interest.
Output of Android Push Notification Example
When we run above example the use of android virtual device (AVD) we are able to get a end result like as proven beneath.
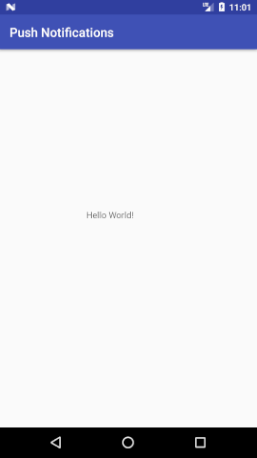
Push Notifications using Firebase Console
To publish or push notifications, we need to go to Firebase Console and open our app through clicking on it. After that, click on on Notifications tab in the left panel. In case, if you go to first time, click on on Send Your First Message otherwise click on New Message.
After that input Message Text, select Target as User Segment then pick your app from the listing and click on Send Message like as proven below.
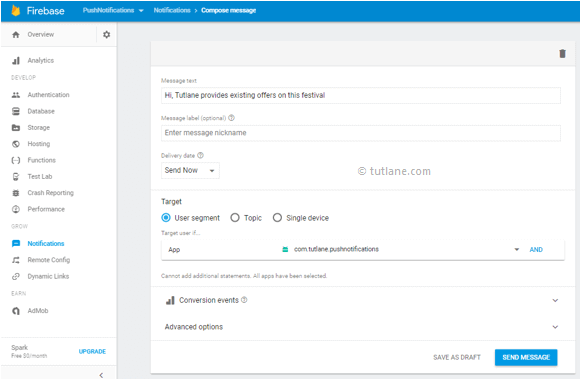
Once we click on Send Message button, we are able to get a notification in our app like as proven below.
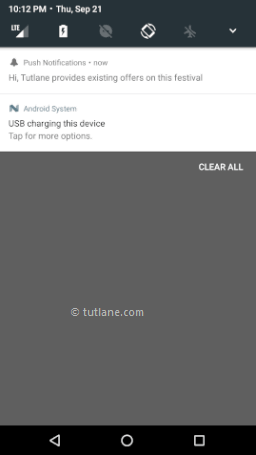
This is how we can push notifications using firebase cloud messaging in android applications based on our requirements.