Fragment(UI),A Fragment is a self-contained issue with its personal person interface (UI) and lifecycle that may be reused in special parts of an app’s UI. (A Fragment also can be used without a UI, so one can retain values throughout configuration modifications, but this lesson does no longer cover that usage.)
A Fragment can be a static part of the UI of an Activity, because of this that the Fragment remains at the screen at some point of the entire lifecycle of the Activity. However, the UI of an Activity can be more effective if it adds or eliminates the Fragment dynamically whilst the Activity is walking.
One instance of a dynamic Fragment is the DatePicker object, that is an example of DialogFragment, a subclass of Fragment. The date picker displays a dialog window floating on top of its Activity window while a user faucets a button or an motion takes place. The consumer can click on OK or Cancel to close the Fragment.
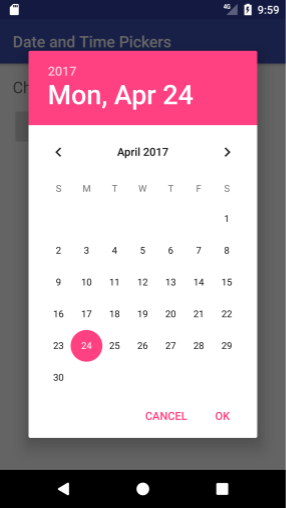
This sensible introduces the Fragment class and suggests you the way to consist of a Fragment as a static a part of a UI, in addition to the way to use Fragment transactions to feature, update, or dispose of a Fragment dynamically.
Fragment(UI) ,What you should already KNOW
You should be able to:
- Create and run apps in Android Studio.
- Use the format editor to create a UI with a ConstraintLayout.
- Inflate the UI layout for an Activity.
- Add a set of radio buttons with a listener to a UI.
Fragment(UI) ,What you will LEARN
You will discover ways to:
- Create a Fragment with an interactive UI.
- Add a Fragment to the format of an Activity.
- Add, update, and cast off a Fragment at the same time as an Activity is going for walks.
Fragment(UI) ,What you will DO
- Create a Fragment to use as a UI detail that offers customers a “yes” or “no” desire.
- Add interactive elements to the Fragment that allow the user to select “yes” or “no”.
- Include the Fragment during an Activity.
- Use Fragment transactions to add, replace, and put off a Fragment whilst an Activity is strolling.
Fragment(UI) ,App overview
Fragment(UI) ,The FragmentExample1 app shows an photo and the title and text of a magazine article. It additionally shows a Fragment that permits users to provide feedback for the item. In this case the comments is very simple: simply “Yes” or “No” to the question “Like the object?” Depending on whether the consumer offers fine or negative comments, the app shows the appropriate reaction.
The Fragment is skeletal, but it demonstrates a way to create a Fragment to use in a couple of places for your app’s UI.
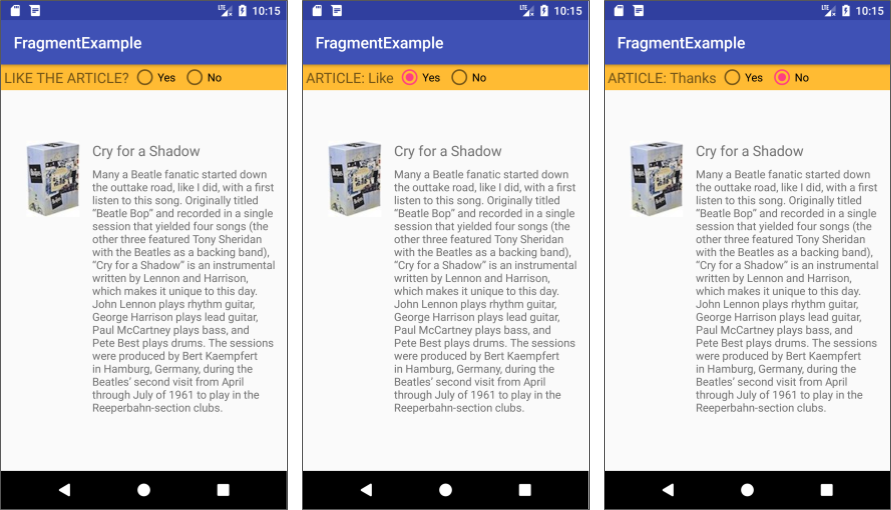
In the first mission, you add the Fragment statically to the Activity layout so that it’s miles displayed for the complete duration of the Activity lifecycle. The consumer can engage with the radio buttons in the Fragment to pick out both “Yes” or “No,” as proven in the discern above.
If the user chooses “Yes,” the text in the Fragment modifications to “Article: Like.” If the consumer chooses “No,” the text within the Fragment modifications to “Article: Thanks.” In the second one venture, in that you create the FragmentExample2 app, you upload the Fragment dynamically — your code provides, replaces, and removes the Fragment even as the Activity is going for walks. You will trade the Activity code and layout to do that.
As proven under, the person can faucet the Open button to show the Fragment at the top of the display. The consumer can then engage with the UI elements inside the Fragment. The user taps Close to shut the Fragment.
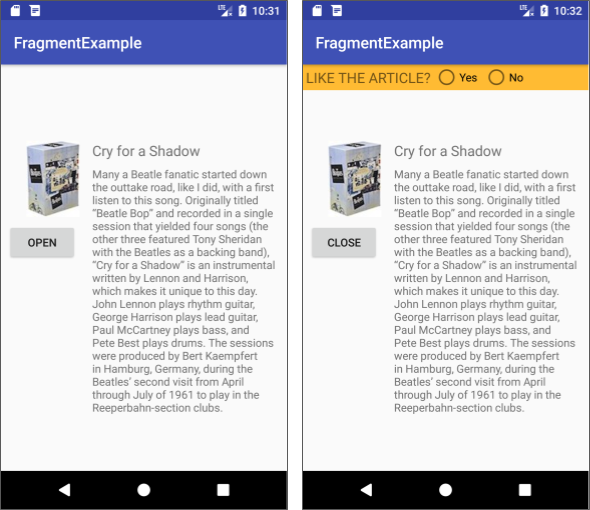
Fragment(UI) ,Include a Fragment for the entire Activity lifecycle
Fragment(UI) ,In this mission you modify a starter app to add a Fragment statically as a part of the layout of the Activity, this means that that the Fragment is shown at some stage in the whole lifecycle of the Activity. This is a beneficial technique for consolidating a set of UI factors (together with radio buttons and textual content) and person interaction behavior that you could reuse in layouts for different sports.
Open the starter app project
Download the FragmentExample_start Android Studio task. Refactor and rename the task to FragmentExample1. (For assist with copying tasks and refactoring and renaming, see Copy and rename a task.) Explore the app the use of Android Studio.
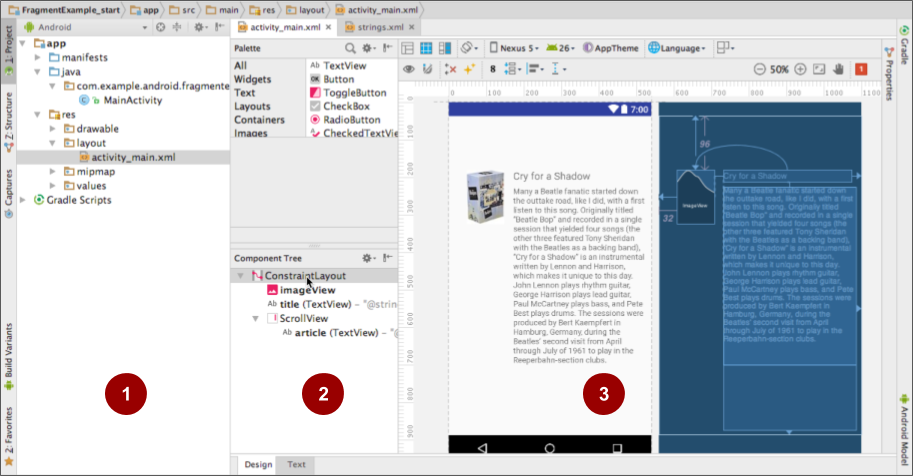
In the above parent:
- The Project: Android pane indicates the contents of the venture.
- The Component Tree pane for the activity_main.Xml layout suggests the android:identity fee of every UI detail.
- The format consists of photo and textual content factors arranged to offer a area at the top for the app bar and a Fragment.
Add a Fragment
- In Project: Android view, make bigger app > java and pick out com.Instance.Android.Fragmentexample.
- Choose File > New > Fragment > Fragment (Blank).
- In the Configure Component conversation, call the Fragment SimpleFragment. The Create layout XML choice have to already be decided on, and the Fragment layout might be crammed in as fragment_simple.
- Uncheck the Include fragment factory techniques and Include interface callbacks options. Click Finish to create the Fragment.
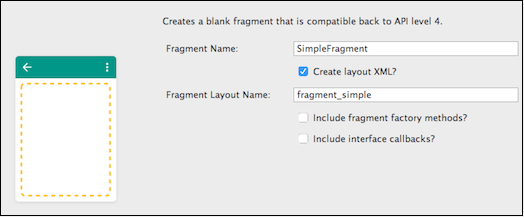
Open SimpleFragment
, and inspect the code:
public class SimpleFragment extends Fragment {
public SimpleFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_simple,
container, false);
All subclasses of Fragment should include a public no-argument constructor as shown. The Android framework often re-instantiates a Fragment item while wished, specially during country repair. The framework needs so that it will locate this constructor so it could instantiate the Fragment.
The Fragment magnificence uses callback techniques which might be just like Activity callback strategies. For instance, onCreateView() presents a LayoutInflater to inflate the Fragment UI from the format aid fragment_simple.
Edit the Fragment’s layout
- Open fragment_simple.Xml. In the layout editor pane, click Text to view the XML.
- Change the attributes for the “Hello blank fragment” TextView:
TextView attribute |
Value |
android:id |
"@+id/fragment_header" |
android:layout_width |
"wrap_content" |
android:layout_height |
"wrap_content" |
android:textAppearance |
"@style/Base.TextAppearance.AppCompat.Medium" |
android:padding |
"4dp" |
android:text |
"@string/question_article" |
The @string/question_article aid is defined inside the strings.Xml record in the starter app as “LIKE THE ARTICLE?”.
- Change the FrameLayout root element to LinearLayout, and exchange the subsequent attributes for the LinearLayout:
LinearLayout attribute |
Value |
android:layout_height |
"wrap_content" |
android:background |
"@color/my_fragment_color" |
android:orientation |
"horizontal" |
The my_fragment_color fee for the android:heritage characteristic is already described inside the starter app in colours.Xml.
- Within the LinearLayout, upload the following RadioGroup element after the TextView element:
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<RadioButton
android:id="@+id/radio_button_yes"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="8dp"
android:text="@string/yes" />
<RadioButton
android:id="@+id/radio_button_no"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="8dp"
android:text="@string/no" />
</RadioGroup>
The @string/sure and @string/no resources are defined inside the strings.Xml record within the starter app as “Yes” and “No”. In addition, the following string sources also are described in the strings.Xml report:
<string name="yes_message">ARTICLE: Like</string>
<string name="no_message">ARTICLE: Thanks</string>
The format preview for fragment_simple.Xml must appear to be the following:
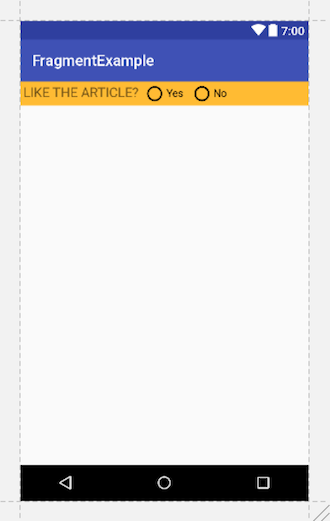
Add a listener for the radio buttons
Add the RadioGroup.OnCheckedChangeListener interface to outline a callback to be invoked while a radio button is checked or unchecked:
- Open SimpleFragment again.
- Change the code in onCreateView() to the code below. This code units up the View and the RadioGroup, and returns the View (rootView). The View and RadioGroup are declared as very last, this means that they may not exchange. This is due to the fact you may want to access them from inside an anonymous inner class (the OnCheckedChangeListener for the RadioGroup):
// Inflate the layout for this fragment.
final View rootView =
inflater.inflate(R.layout.fragment_simple, container, false);
final RadioGroup radioGroup = rootView.findViewById(R.id.radio_group);
// TODO: Set the radioGroup onCheckedChanged listener.
// Return the View for the fragment's UI.
return rootView;
Add the following constants to the pinnacle of SimpleFragment to symbolize the two states of the radio button desire: 0 = sure and 1 = no:
private static final int YES = 0;
private static final int NO = 1;
- Replace the TODO comment within the onCreateView() approach with the following code to set the radio organization listener and exchange the textView (the fragment_header within the layout) relying at the radio button desire:
radioGroup.setOnCheckedChangeListener(new
RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
View radioButton = radioGroup.findViewById(checkedId);
int index = radioGroup.indexOfChild(radioButton);
TextView textView =
rootView.findViewById(R.id.fragment_header);
switch (index) {
case YES: // User chose "Yes."
textView.setText(R.string.yes_message);
break;
case NO: // User chose "No."
textView.setText(R.string.no_message);
break;
default: // No choice made.
// Do nothing.
break;
}
}
});
- The onCheckedChanged() approach must be carried out for RadioGroup.OnCheckedChangeListener; in this case, the index for the two radio buttons is zero (“Yes”) or 1 (“No”), which display either the yes_message text or the no_message text.
Add the Fragment statically to the Activity
- Open activity_main.Xml.
- Add the following detail underneath the ScrollView within the ConstraintLayout root view. It refers to the SimpleFragment you simply created:
<fragment
android:id="@+id/fragment"
android:name="com.example.android.fragmentexample.SimpleFragment"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:layout="@layout/fragment_simple" />
The figure below shows the layout preview, with SimpleFragment
statically included in the activity layout:
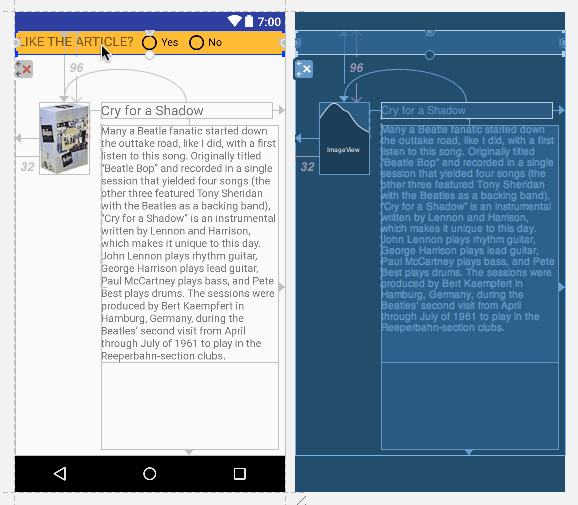
A render errors may additionally appear underneath the preview, because a element can dynamically include distinct layouts while the app runs, and the format editor does not realize what format to use for a preview. To see the Fragment in the preview, click the @format/fragment_simple link inside the errors message, and pick out SimpleFragment.
- Run the app. The Fragment is now protected in the MainActivity format, as proven inside the figures beneath.
- Tap a radio button. The “LIKE THE ARTICLE?” textual content is changed by way of either the yes_message text (“ARTICLE: Like”) or the no_message text (“ARTICLE: Thanks”), depending on which radio button is tapped.
- After tapping a radio button, change the orientation of your tool or emulator from portrait to panorama. Note that the “Yes” or “No” preference continues to be decided on.
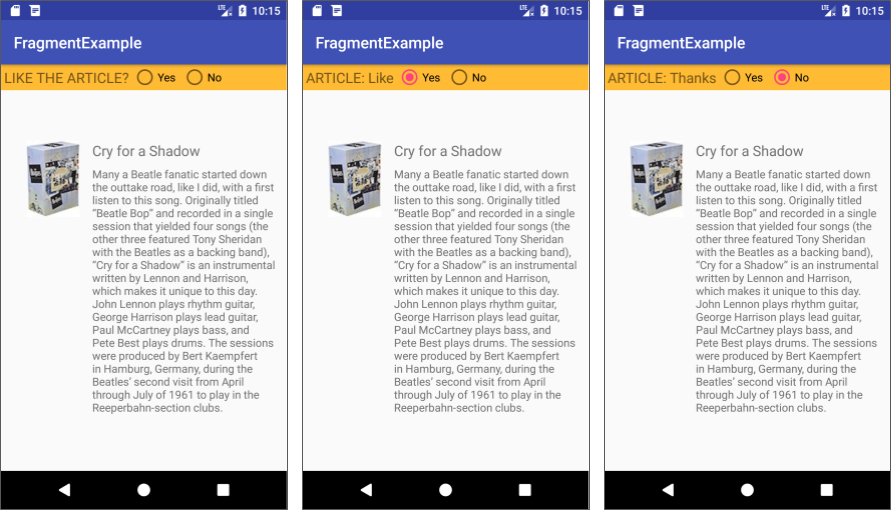
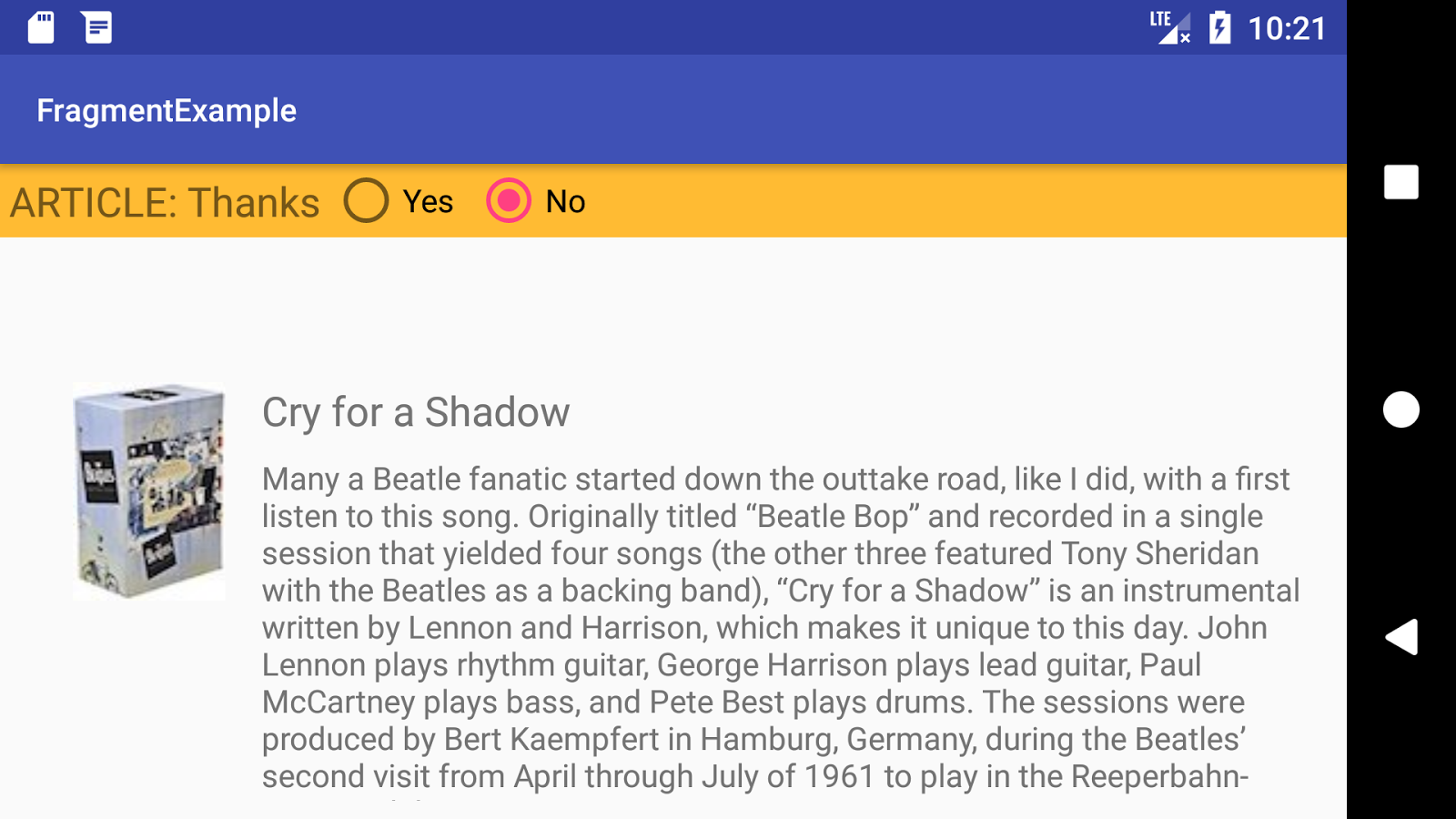
Switching the device orientation after deciding on “No” demonstrates that a Fragment can preserve an example of its records after a configuration exchange (including changing the orientation). This characteristic makes a Fragment useful as a UI component, in comparison to the usage of separate Views. While an Activity is destroyed and recreated when a device’s configuration modifications, a Fragment isn’t destroyed.
Note: All coding challenges are optional.
Challenge:Expand the Fragment to consist of some other query (“Like the song?”) under the primary question, so that it appears as shown inside the parent under. Add a RatingBar so that the user can set a score for the tune, and a Toast message that shows the chosen score.
Hint:Use the OnRatingBarChangeListener in the Fragment, and make sure to consist of the android:isIndicator characteristic, set to fake, for the RatingBar within the Fragment layout.
This challenge demonstrates how a Fragment
can handle different kinds of user input.
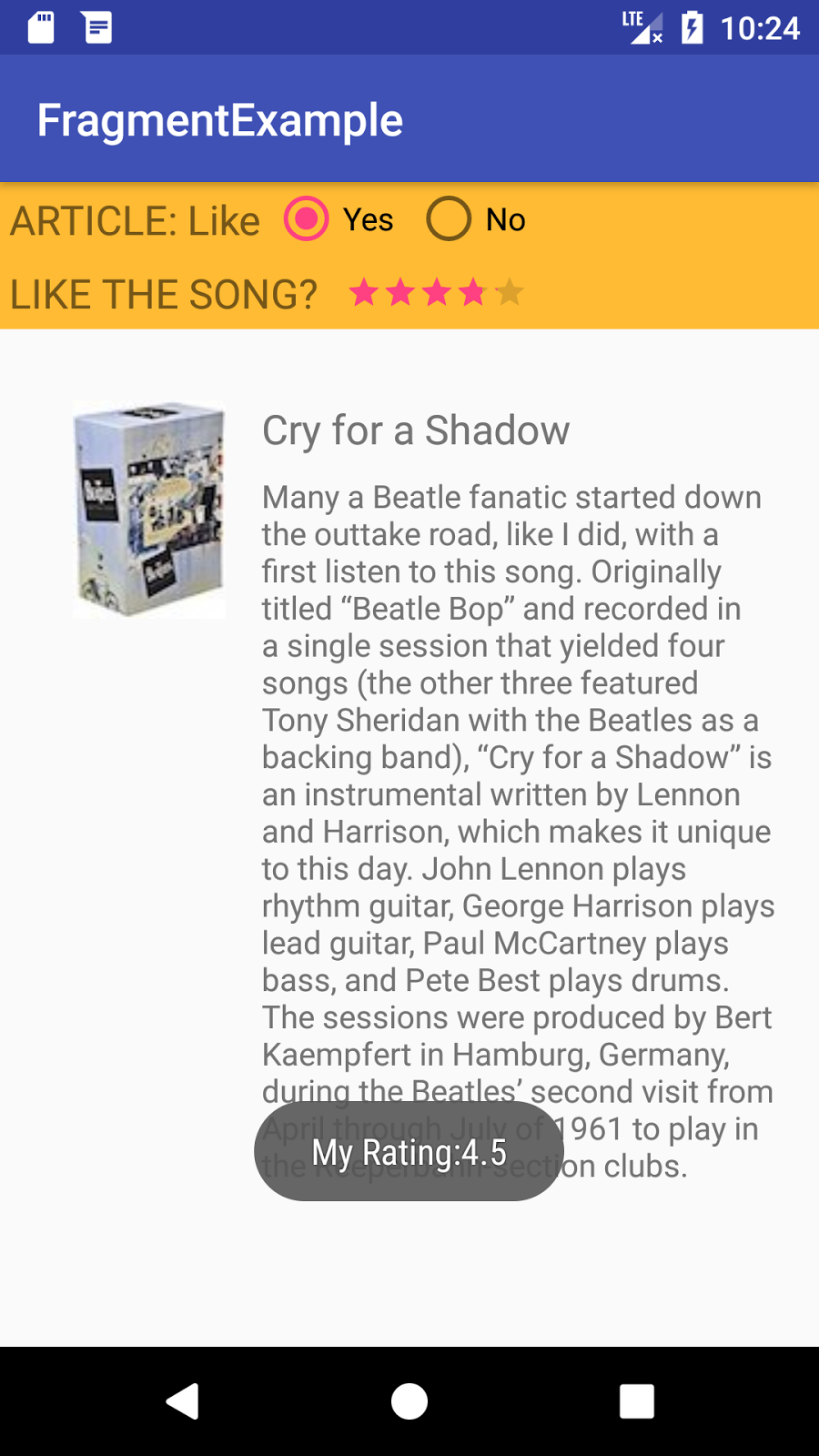
Add a Fragment to an Activity dynamically
In this task you discover ways to add the same Fragment to an Activity dynamically . The Fragment is added simplest if the user performs an interplay inside the Activity—in this example, tapping a button.
You will alternate the FragmentExample1 app to manipulate the Fragment using FragmentManager and FragmentTransaction statements which can upload, remove, and update a Fragment.
Add a ViewGroup for the Fragment
At any time at the same time as your Activity is jogging, your code can add a Fragment to the Activity. All your Activity code wishes is a ViewGroup in the layout as a placeholder for the Fragment, together with a FrameLayout. Change the detail to within the fundamental layout. Follow these steps:
- Copy the FragmentExample1 venture, and open the replica in Android Studio. Refactor and rename the undertaking to FragmentExample2. (For help with copying projects and refactoring and renaming, see Copy and rename a task.)
- If a caution seems to cast off the FragmentExample1 modules, click to dispose of them
- Open the activity_main.Xml format, and switch the layout editor to Text (XML) view.
- Change the element to , and change the android:identification to fragment_container, as proven under:
<FrameLayout
android:id="@+id/fragment_container"
Add a button to open and close the Fragment
Users need a manner to open and close the Fragment from the Activity. To maintain this situation simple, upload a Button to open the Fragment, and if the Fragment is open, the same Button can near the Fragment.
- Open activity_main.Xml, click the Design tab if it is not already selected, and upload a Button below the imageView element.
- Constrain the Button to the bottom of imageView and to the left side of the discern.
- Open the Attributes pane, and add the ID open_button and the text “@string/open”. The @string/open and @string/close sources are described in the strings.Xml record in the starter app as “OPEN” and “CLOSE”.
Note that due to the fact you have modified to , the Fragment (now referred to as fragment_container) now not seems inside the layout preview—but do not worry, it’s still there!
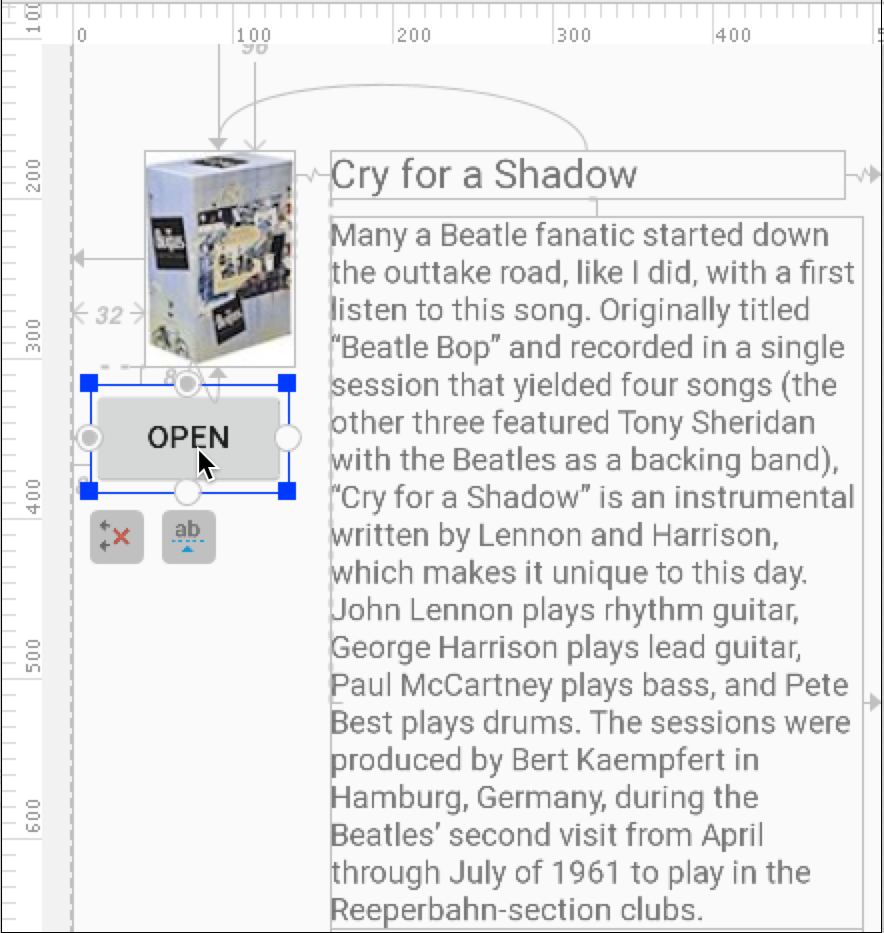
- Open MainActivity, and declare and initialize the Button. Also upload a non-public boolean to decide whether or not the Fragment is displayed:
private Button mButton;
private boolean isFragmentDisplayed = false;
- Add the following to the onCreate() approach to initialize the mButton:
mButton = findViewById(R.id.open_button);
Use Fragment transactions
To manipulate a Fragment to your Activity, use FragmentManager. To carry out a Fragment transaction to your Activity—including including, getting rid of, or changing a Fragment—use methods in FragmentTransaction.
The pleasant practice for instantiating the Fragment inside the Activity is to offer a newinstance() manufacturing unit technique within the Fragment. Follow those steps to add the newinstance() technique to SimpleFragment and instantiate the Fragment in MainActivity. You will also add the displayFragment() and closeFragment() methods, and use Fragment transactions:
- Open SimpleFragment, and upload the subsequent technique for instantiating and returning the Fragment to the Activity:
public static SimpleFragment newInstance() {
return new SimpleFragment();
}
- Open MainActivity, and create the following displayFragment() approach to instantiate and open SimpleFragment. It starts through developing an instance of simpleFragment by using calling the newInstance() technique in SimpleFragment:
public void displayFragment() {
SimpleFragment simpleFragment = SimpleFragment.newInstance();
// TODO: Get the FragmentManager and start a transaction.
// TODO: Add the SimpleFragment.
}
- Replace the TODO: Get the FragmentManager… Remark in the above code with the subsequent:
// Get the FragmentManager and start a transaction.
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager
.beginTransaction();
To start a transaction, get an example of FragmentManager the use of getSupportFragmentManager(), after which get an instance of FragmentTransaction that uses beginTransaction(). Fragment operations are wrapped into a transaction (much like a financial institution transaction) so that each one the operations finish before the transaction is dedicated for the very last end result.
Use getSupportFragmentManager() (instead of getFragmentManager()) to instantiate the Fragment elegance so your app remains well suited with gadgets walking system versions as little as Android 1.6. (The getSupportFragmentManager() technique uses the Support Library.)
- Replace the TODO: Add the SimpleFragment remark inside the above code with the following:
// Add the SimpleFragment.
fragmentTransaction.add(R.id.fragment_container,
simpleFragment).addToBackStack(null).commit();
// Update the Button text.
mButton.setText(R.string.close);
// Set boolean flag to indicate fragment is open.
isFragmentDisplayed = true;
This code adds a new Fragment the usage of the add() transaction approach. The first argument handed to add() is the format aid (fragment_container) for the ViewGroup wherein the Fragment should be positioned. The 2nd parameter is the Fragment (simpleFragment) to feature.
In addition to the upload() transaction, the code calls addToBackStack(null) to be able to add the transaction to a back stack of Fragment transactions. This lower back stack is managed through the Activity. It allows the user to go back to the previous Fragment country through urgent the Back button.
The code then calls commit() for the transaction to take effect.
The code also modifications the textual content of the Button to “CLOSE” and sets the Boolean isFragmentDisplayed to real so that you can music the country of the Fragment.
- To near the Fragment, add the subsequent closeFragment() technique to MainActivity:
public void closeFragment() {
// Get the FragmentManager.
FragmentManager fragmentManager = getSupportFragmentManager();
// Check to see if the fragment is already showing.
SimpleFragment simpleFragment = (SimpleFragment) fragmentManager
.findFragmentById(R.id.fragment_container);
if (simpleFragment != null) {
// Create and commit the transaction to remove the fragment.
FragmentTransaction fragmentTransaction =
fragmentManager.beginTransaction();
fragmentTransaction.remove(simpleFragment).commit();
}
// Update the Button text.
mButton.setText(R.string.open);
// Set boolean flag to indicate fragment is closed.
isFragmentDisplayed = false;
}
As with displayFragment(), the closeFragment() code snippet receives an instance of FragmentManager the use of getSupportFragmentManager(), makes use of beginTransaction() to begin a series of transactions, and acquires a connection with the Fragment the usage of the layout aid (fragment_container). It then uses the remove() transaction to remove the Fragment.
However, earlier than growing this transaction, the code checks to peer if the Fragment is displayed (now not null). If the Fragment is not displayed, there may be not anything to dispose of.
The code also changes the textual content of the Button to “OPEN” and units the Boolean isFragmentDisplayed to fake so you can track the country of the Fragment.
Set the Button onClickListener
To take action while the user clicks the Button, implement an OnClickListener for the button inside the onCreate() technique of MainActivity if you want to open and close the fragment based on the boolean price of isFragmentDisplayed. The onClick() technique will name the displayFragment() approach to open the Fragment if the Fragment isn’t already open; in any other case it calls closeFragment().
You will also upload code to store the value of isFragmentDisplayed and use it if the configuration changes, such as if the user switches from portrait or landscape orientation.
- Open MainActivity, and upload the subsequent to the onCreate() approach to set the click listener for the Button:
// Set the click listener for the button.
mButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (!isFragmentDisplayed) {
displayFragment();
} else {
closeFragment();
}
}
});
- To shop the boolean cost representing the Fragment show nation, outline a key for the Fragment state to apply in the savedInstanceState Bundle. Add this member variable to MainActivity:
static final String STATE_FRAGMENT = "state_of_fragment";
- Add the subsequent approach to MainActivity to keep the country of the Fragment if the configuration modifications:
public void onSaveInstanceState(Bundle savedInstanceState) {
// Save the state of the fragment (true=open, false=closed).
savedInstanceState.putBoolean(STATE_FRAGMENT, isFragmentDisplayed);
super.onSaveInstanceState(savedInstanceState);
}
- Go returned to the onCreate() technique and add the following code. It assessments to look if the instance nation of the Activity turned into saved for a few cause, inclusive of a configuration trade (the user switching from vertical to horizontal). If the stored instance was not stored, it might be null. If the stored instance isn’t always null, the code retrieves the Fragment nation from the saved instance, and sets the Button textual content:
if (savedInstanceState != null) {
isFragmentDisplayed =
savedInstanceState.getBoolean(STATE_FRAGMENT);
if (isFragmentDisplayed) {
// If the fragment is displayed, change button to "close".
mButton.setText(R.string.close);
}
}
- Run the app. Tapping Open adds the Fragment and suggests the Close textual content in the button. Tapping Close eliminates the Fragment and suggests the Open text in the button. You can switch your device or emulator from vertical to horizontal orientation to see that the buttons and Fragment paintings.
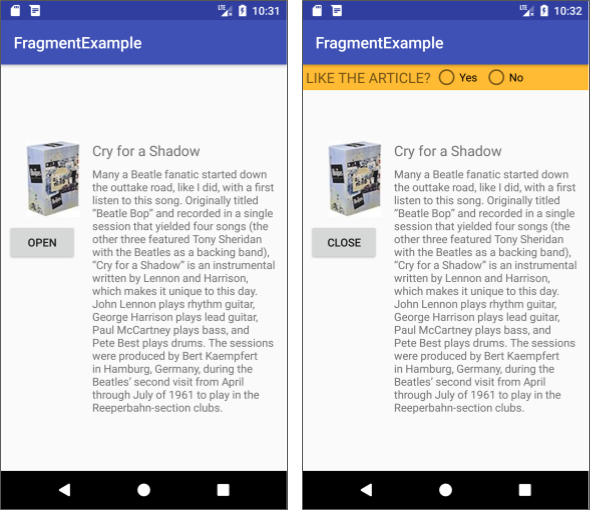
Summary
- To create a clean Fragment, make bigger app > java in Project: Android view, choose the folder containing the Java code for your app, and pick out File > New > Fragment > Fragment (Blank).
- The Fragment magnificence makes use of callback techniques that are just like Activity callbacks, along with onCreateView(), which presents a LayoutInflater object to inflate the Fragment UI from the layout resource fragment_simple.
- To upload a Fragment statically to an Activity so that it’s miles displayed for the complete lifecycle of the Activity, claim the Fragment inside the layout file for the Activity using the tag. You can specify layout attributes for the Fragment as though it had been a View.
- To add a Fragment dynamically to an Activity so that the Activity can add, update, or put off it, specify a ViewGroup inside the layout record for the Activity which include a FrameLayout.
- When including a Fragment dynamically to an Activity, the satisfactory exercise for growing the fragment is to create the example with a newinstance() method in the Fragment itself. Call the newinstance() technique from the Activity to create a new example.
- To get an example of FragmentManager, use getSupportFragmentManager() to be able to instantiate the Fragment elegance the usage of the Support Library so your app stays well matched with gadgets jogging gadget versions as little as Android 1.6.
- To start a chain of Fragment transactions, name beginTransaction() onaFragmentTransaction.
- With FragmentManager your code can carry out the following Fragment transactions even as the app runs, the usage of FragmentTransaction methods:
Add a Fragment the usage of upload(). Remove a Fragment the use of cast off(). Replace a Fragment with any other Fragment the usage of update().