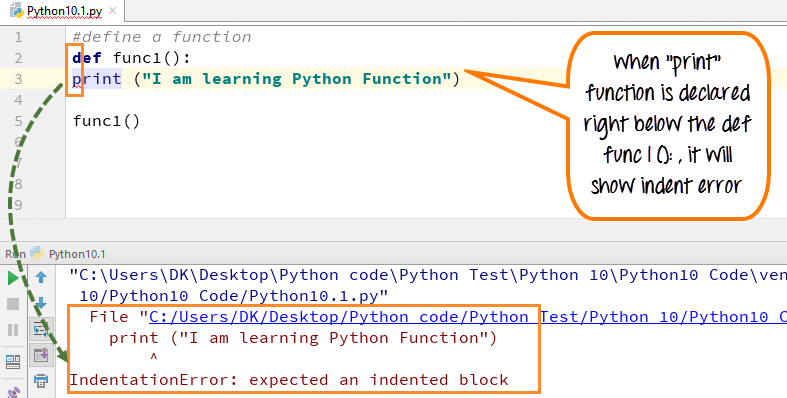
Python – Functions, A characteristic is a block of organized, reusable code that is used to carry out a single, related motion.
Functions offer higher modularity in your utility and a excessive diploma of code reusing.
As you understand, Python gives you many integrated functions like print(), and so forth. But you can also create your own features. These functions are known as consumer-described features.
Defining a Function
You can outline capabilities to provide the desired functionality. Here are easy regulations to outline a function in Python.
- Function blocks start with the keyword def observed by using the feature call and parentheses ( ( ) ).
- Any enter parameters or arguments should be positioned inside those parentheses. You also can define parameters inside these parentheses.
- The first declaration of a function may be an optionally available assertion – the documentation string of the characteristic or docstring.
- The code block within every function starts with a colon (:) and is indented.
- The assertion go back [expression] exits a function, optionally passing back an expression to the caller. A go back announcement with no arguments is similar to go back None.
Syntax
def functionname( parameters ):
"function_docstring"
function_suite
return [expression]
By default, parameters have a positional behavior and you want to inform them inside the identical order that they have been described.
Example
The following function takes a string as enter parameter and prints it on widespread display.
def printme( str ):
"This prints a passed string into this function"
print str
return
Calling a Function
Defining a function handiest offers it a call, specifies the parameters which can be to be blanketed within the function and systems the blocks of code.
Once the simple structure of a feature is finalized, you could execute it through calling.
It from some other feature or directly from the Python prompt. Following is the example to call printme() feature −
#!/usr/bin/python
# Function definition is here
def printme( str ):
"This prints a passed string into this function"
print str
return;
# Now you can call printme function
printme("I'm first call to user defined function!")
printme("Again second call to the same function")
When the above code is achieved, it produces the subsequent result −
I'm first call to user defined function!
Again second call to the same function
Pass by reference vs value
All parameters (arguments) in the Python language are passed by reference. It way if you trade what a parameter refers to within a feature.
The change additionally displays returned within the calling function. For instance −
#!/usr/bin/python
# Function definition is here
def changeme( mylist ):
"This changes a passed list into this function"
mylist.append([1,2,3,4]);
print "Values inside the function: ", mylist
return
# Now you can call changeme function
mylist = [10,20,30];
changeme( mylist );
print "Values outside the function: ", mylist
Here, we’re retaining reference of the exceeded item and appending values in the same item. So, this would produce the following result −
Values inside the function: [10, 20, 30, [1, 2, 3, 4]]
Values outside the function: [10, 20, 30, [1, 2, 3, 4]]
There is one more example in which argument is being passed by way of reference and the reference is being overwritten within the known as characteristic.
#!/usr/bin/python
# Function definition is here
def changeme( mylist ):
"This changes a passed list into this function"
mylist = [1,2,3,4]; # This would assig new reference in mylist
print "Values inside the function: ", mylist
return
# Now you can call changeme function
mylist = [10,20,30];
changeme( mylist );
print "Values outside the function: ", mylist
The parameter mylist is neighborhood to the characteristic changeme. Changing mylist inside the feature does now not affect mylist.
The function accomplishes not anything and sooner or later this will produce the following result −
Values inside the function: [1, 2, 3, 4]
Values outside the function: [10, 20, 30]
Python – Functions, Function Arguments
You can name a feature with the aid of using the subsequent sorts of formal arguments −
- Required arguments
- Keyword arguments
- Default arguments
- Variable-duration arguments
Python – Functions, Required arguments
Required arguments are the arguments surpassed to a feature in accurate positional order.
Here, the wide variety of arguments in the feature name ought to match exactly with the function definition.
To name the feature printme(), you clearly need to bypass one argument, otherwise it offers a syntax mistakes as follows −
#!/usr/bin/python
# Function definition is here
def printme( str ):
"This prints a passed string into this function"
print str
return;
# Now you can call printme function
printme()
When the above code is done, it produces the following end result −
Traceback (most recent call last):
File "test.py", line 11, in <module>
printme();
TypeError: printme() takes exactly 1 argument (0 given)
Python – Functions, Keyword arguments
Keyword arguments are associated with the function calls. When you operate key-word arguments in a feature name, the caller identifies the arguments by means of the parameter name.
This allows you to pass arguments or location them out of order due to the fact the Python interpreter is able to use the keywords furnished to suit.
The values with parameters. You also can make keyword calls to the printme() feature in the following approaches −
#!/usr/bin/python
# Function definition is here
def printme( str ):
"This prints a passed string into this function"
print str
return;
# Now you can call printme function
printme( str = "My string")
When the above code is done, it produces the subsequent result −
My string
The following example gives extra clear picture. Note that the order of parameters does now not count number.
#!/usr/bin/python
# Function definition is here
def printinfo( name, age ):
"This prints a passed info into this function"
print "Name: ", name
print "Age ", age
return;
# Now you can call printinfo function
printinfo( age=50, name="miki" )
When the above code is executed, it produces the following end result −
Name: miki
Age 50
Python – Functions, Default arguments
A default argument is a controversy that assumes a default fee if a value isn’t always provided inside.
The function name for that argument. The following instance offers an concept on default arguments, it prints default age if it isn’t always exceeded −
#!/usr/bin/python
# Function definition is here
def printinfo( name, age = 35 ):
"This prints a passed info into this function"
print "Name: ", name
print "Age ", age
return;
# Now you can call printinfo function
printinfo( age=50, name="miki" )
printinfo( name="miki" )
When the above code is executed, it produces the following result −
Name: miki
Age 50
Name: miki
Age 35
Python – Functions, Variable-length arguments
You may additionally want to process a feature for greater arguments than you exact whilst defining the function. These arguments are referred to as variable-duration.
Arguments and are not named inside the characteristic definition, in contrast to required and default arguments.
Syntax for a feature with non-keyword variable arguments is that this −
def functionname([formal_args,] *var_args_tuple ):
"function_docstring"
function_suite
return [expression]
An asterisk (*) is placed earlier than the variable call that holds the values of all nonkeyword variable arguments. This tuple remains empty if no extra arguments are specified for the duration of the function name. Following is a simple example −
#!/usr/bin/python
# Function definition is here
def printinfo( arg1, *vartuple ):
"This prints a variable passed arguments"
print "Output is: "
print arg1
for var in vartuple:
print var
return;
# Now you can call printinfo function
printinfo( 10 )
printinfo( 70, 60, 50 )
When the above code is executed, it produces the following result −
Output is:
10
Output is:
70
60
50
Python – Functions, The Anonymous Functions
These functions are referred to as nameless due to the fact they are no longer declared inside.
The general manner via the usage of the def keyword. You can use the lambda keyword to create small nameless features.
- Lambda forms can take any quantity of arguments however go back just one price within the shape of an expression. They cannot incorporate commands or multiple expressions.
- An anonymous function can’t be an instantaneous name to print due to the fact lambda requires an expression
- Lambda capabilities have their very own nearby namespace and cannot get right of entry to variables other than those of their parameter list and those inside the worldwide namespace.
- Although it appears that lambda’s are a one-line model of a function, they are not equal to inline statements in C or C++, whose purpose is by means of passing function stack allocation throughout invocation for overall performance motives.
Syntax
The syntax of lambda functions carries best a single assertion, which is as follows −
lambda [arg1 [,arg2,.....argn]]:expression
Following is the example to show how lambda form of function works −
#!/usr/bin/python
# Function definition is here
sum = lambda arg1, arg2: arg1 + arg2;
# Now you can call sum as a function
print "Value of total : ", sum( 10, 20 )
print "Value of total : ", sum( 20, 20 )
When the above code is done, it produces the following result −
Value of total : 30
Value of total : 40
Python – Functions, The return Statement
The statement return [expression] exits a function, optionally passing back an expression to the caller. A return statement with no arguments is the same as return None.
Python – Functions, All the above examples are not returning any value. You can return a value from a function as follows −
#!/usr/bin/python
# Function definition is here
def sum( arg1, arg2 ):
# Add both the parameters and return them."
total = arg1 + arg2
print "Inside the function : ", total
return total;
# Now you can call sum function
total = sum( 10, 20 );
print "Outside the function : ", total
When the above code is executed, it produces the following result −
Inside the function : 30
Outside the function : 30
Python – Functions, Scope of Variables
Python – Functions, All variables in a program won’t be on hand in any respect places in that program. This relies upon on in which you have declared a variable.
The scope of a variable determines the portion of this system where you can get right of entry to a selected identifier. There are two simple scopes of variables in Python −
- Global variables
- Local variables
Python – Functions, Global vs. Local variables
Python – Functions, Variables that are defined interior a characteristic frame have a nearby scope, and those defined outdoor have a worldwide scope.
This manner that neighborhood variables can be accessed only inside the feature wherein they’re declared.
While international variables may be accessed during.
This system body through all features. When you name a characteristic, the variables declared inner it are added into scope. Following is a simple example −
#!/usr/bin/python
total = 0; # This is global variable.
# Function definition is here
def sum( arg1, arg2 ):
# Add both the parameters and return them."
total = arg1 + arg2; # Here total is local variable.
print "Inside the function local total : ", total
return total;
# Now you can call sum function
sum( 10, 20 );
print "Outside the function global total : ", total
When the above code is executed, it produces the following result −
Inside the function local total : 30
Outside the function global total : 0